mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
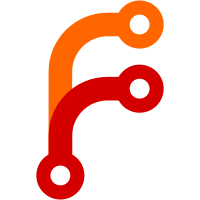
Due to some recent optimizations related to the compare instruction, DS-form load instructions started to be used to load 8-byte go.strings. This can cause link time errors if the go.string is not aligned to 4 bytes. For DS-form instructions, the value in the offset field must be a multiple of 4. If the offset is known at the time the rules are processed, a DS-form load will not be chosen. But for go.strings, the offset is not known at that time, but a relocation is generated indicating that the linker should fill in the DS relocation. When the linker tries to fill in the relocation, if the offset is not aligned properly, a link error will occur. To fix this, when loading a go.string using MOVDload, the full address of the go.string is generated and loaded into the base register. Then the go.string is loaded with a 0 offset field. Added a testcase that reproduces this problem. Fixes #24799 Change-Id: I6a154e8e1cba64eae290be0fbcb608b75884ecdd Reviewed-on: https://go-review.googlesource.com/107855 Run-TryBot: Lynn Boger <laboger@linux.vnet.ibm.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: David Chase <drchase@google.com>
58 lines
1.4 KiB
Go
58 lines
1.4 KiB
Go
// run
|
|
|
|
// Copyright 2018 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Loads of 8 byte go.strings cannot use DS relocation
|
|
// in case the alignment is not a multiple of 4.
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
)
|
|
|
|
type Level string
|
|
|
|
// The following are all go.strings. A link time error can
|
|
// occur if an 8 byte load is used to load a go.string that is
|
|
// not aligned to 4 bytes due to the type of relocation that
|
|
// is generated for the instruction. A fix was made to avoid
|
|
// generating an instruction with DS relocation for go.strings
|
|
// since their alignment is not known until link time.
|
|
|
|
// This problem only affects go.string since other types have
|
|
// correct alignment.
|
|
|
|
const (
|
|
LevelBad Level = "badvals"
|
|
LevelNone Level = "No"
|
|
LevelMetadata Level = "Metadata"
|
|
LevelRequest Level = "Request"
|
|
LevelRequestResponse Level = "RequestResponse"
|
|
)
|
|
|
|
func ordLevel(l Level) int {
|
|
switch l {
|
|
case LevelMetadata:
|
|
return 1
|
|
case LevelRequest:
|
|
return 2
|
|
case LevelRequestResponse:
|
|
return 3
|
|
default:
|
|
return 0
|
|
}
|
|
}
|
|
|
|
//go:noinline
|
|
func test(l Level) {
|
|
if ordLevel(l) < ordLevel(LevelMetadata) {
|
|
fmt.Printf("OK\n")
|
|
}
|
|
}
|
|
|
|
func main() {
|
|
test(LevelMetadata)
|
|
}
|