mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
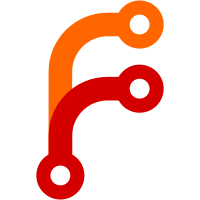
Allow shifts by signed amounts. Panic if the shift amount is negative. TODO: We end up doing two compares per shift, see Ian's comment https://github.com/golang/go/issues/19113#issuecomment-443241799 that we could do it with a single comparison in the normal case. The prove pass mostly handles this code well. For instance, it removes the <0 check for cases like this: if s >= 0 { _ = x << s } _ = x << len(a) This case isn't handled well yet: _ = x << (y & 0xf) I'll do followon CLs for unhandled cases as needed. Update #19113 R=go1.13 Change-Id: I839a5933d94b54ab04deb9dd5149f32c51c90fa1 Reviewed-on: https://go-review.googlesource.com/c/158719 Run-TryBot: Keith Randall <khr@golang.org> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Josh Bleecher Snyder <josharian@gmail.com>
108 lines
1.8 KiB
Go
108 lines
1.8 KiB
Go
// run
|
|
|
|
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import "reflect"
|
|
|
|
var tests = []interface{}{
|
|
func(x int, s int) int {
|
|
return x << s
|
|
},
|
|
func(x int, s int64) int {
|
|
return x << s
|
|
},
|
|
func(x int, s int32) int {
|
|
return x << s
|
|
},
|
|
func(x int, s int16) int {
|
|
return x << s
|
|
},
|
|
func(x int, s int8) int {
|
|
return x << s
|
|
},
|
|
func(x int, s int) int {
|
|
return x >> s
|
|
},
|
|
func(x int, s int64) int {
|
|
return x >> s
|
|
},
|
|
func(x int, s int32) int {
|
|
return x >> s
|
|
},
|
|
func(x int, s int16) int {
|
|
return x >> s
|
|
},
|
|
func(x int, s int8) int {
|
|
return x >> s
|
|
},
|
|
func(x uint, s int) uint {
|
|
return x << s
|
|
},
|
|
func(x uint, s int64) uint {
|
|
return x << s
|
|
},
|
|
func(x uint, s int32) uint {
|
|
return x << s
|
|
},
|
|
func(x uint, s int16) uint {
|
|
return x << s
|
|
},
|
|
func(x uint, s int8) uint {
|
|
return x << s
|
|
},
|
|
func(x uint, s int) uint {
|
|
return x >> s
|
|
},
|
|
func(x uint, s int64) uint {
|
|
return x >> s
|
|
},
|
|
func(x uint, s int32) uint {
|
|
return x >> s
|
|
},
|
|
func(x uint, s int16) uint {
|
|
return x >> s
|
|
},
|
|
func(x uint, s int8) uint {
|
|
return x >> s
|
|
},
|
|
}
|
|
|
|
func main() {
|
|
for _, t := range tests {
|
|
runTest(reflect.ValueOf(t))
|
|
}
|
|
}
|
|
|
|
func runTest(f reflect.Value) {
|
|
xt := f.Type().In(0)
|
|
st := f.Type().In(1)
|
|
|
|
for _, x := range []int{1, 0, -1} {
|
|
for _, s := range []int{-99, -64, -63, -32, -31, -16, -15, -8, -7, -1, 0, 1, 7, 8, 15, 16, 31, 32, 63, 64, 99} {
|
|
args := []reflect.Value{
|
|
reflect.ValueOf(x).Convert(xt),
|
|
reflect.ValueOf(s).Convert(st),
|
|
}
|
|
if s < 0 {
|
|
shouldPanic(func() {
|
|
f.Call(args)
|
|
})
|
|
} else {
|
|
f.Call(args) // should not panic
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
func shouldPanic(f func()) {
|
|
defer func() {
|
|
if recover() == nil {
|
|
panic("did not panic")
|
|
}
|
|
}()
|
|
f()
|
|
}
|