mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
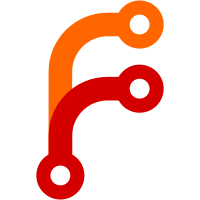
Fixes #18808. Change-Id: I49b266380b9d6804c9f6563ebac9c7c0e05f37f6 Reviewed-on: https://go-review.googlesource.com/35890 Run-TryBot: Michael Munday <munday@ca.ibm.com> Reviewed-by: Cherry Zhang <cherryyz@google.com>
63 lines
847 B
Go
63 lines
847 B
Go
// run
|
|
|
|
// Copyright 2017 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
const lim = 0x80000000
|
|
|
|
//go:noinline
|
|
func eq(x uint32) {
|
|
if x == lim {
|
|
return
|
|
}
|
|
panic("x == lim returned false")
|
|
}
|
|
|
|
//go:noinline
|
|
func neq(x uint32) {
|
|
if x != lim {
|
|
panic("x != lim returned true")
|
|
}
|
|
}
|
|
|
|
//go:noinline
|
|
func gt(x uint32) {
|
|
if x > lim {
|
|
return
|
|
}
|
|
panic("x > lim returned false")
|
|
}
|
|
|
|
//go:noinline
|
|
func gte(x uint32) {
|
|
if x >= lim {
|
|
return
|
|
}
|
|
panic("x >= lim returned false")
|
|
}
|
|
|
|
//go:noinline
|
|
func lt(x uint32) {
|
|
if x < lim {
|
|
panic("x < lim returned true")
|
|
}
|
|
}
|
|
|
|
//go:noinline
|
|
func lte(x uint32) {
|
|
if x <= lim {
|
|
panic("x <= lim returned true")
|
|
}
|
|
}
|
|
|
|
func main() {
|
|
eq(lim)
|
|
neq(lim)
|
|
gt(lim+1)
|
|
gte(lim+1)
|
|
lt(lim+1)
|
|
lte(lim+1)
|
|
}
|