mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
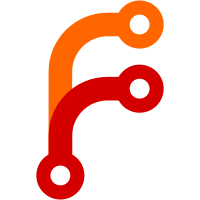
Following CL 357330, use jump tables on ARM64. name old time/op new time/op delta Switch8Predictable-4 3.41ns ± 0% 3.21ns ± 0% ~ (p=0.079 n=4+5) Switch8Unpredictable-4 12.0ns ± 0% 9.5ns ± 0% -21.17% (p=0.000 n=5+4) Switch32Predictable-4 3.06ns ± 0% 2.82ns ± 0% -7.78% (p=0.008 n=5+5) Switch32Unpredictable-4 13.3ns ± 0% 9.5ns ± 0% -28.87% (p=0.016 n=4+5) SwitchStringPredictable-4 3.71ns ± 0% 3.21ns ± 0% -13.43% (p=0.000 n=5+4) SwitchStringUnpredictable-4 14.8ns ± 0% 15.1ns ± 0% +2.37% (p=0.008 n=5+5) Change-Id: Ia0b85df7ca9273cf70c05eb957225c6e61822fa6 Reviewed-on: https://go-review.googlesource.com/c/go/+/403979 TryBot-Result: Gopher Robot <gobot@golang.org> Reviewed-by: Keith Randall <khr@golang.org> Run-TryBot: Cherry Mui <cherryyz@google.com> Reviewed-by: David Chase <drchase@google.com>
74 lines
1.1 KiB
Go
74 lines
1.1 KiB
Go
// asmcheck
|
|
|
|
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// These tests check code generation of switch statements.
|
|
|
|
package codegen
|
|
|
|
// see issue 33934
|
|
func f(x string) int {
|
|
// amd64:-`cmpstring`
|
|
switch x {
|
|
case "":
|
|
return -1
|
|
case "1", "2", "3":
|
|
return -2
|
|
default:
|
|
return -3
|
|
}
|
|
}
|
|
|
|
// use jump tables for 8+ int cases
|
|
func square(x int) int {
|
|
// amd64:`JMP\s\(.*\)\(.*\)$`
|
|
// arm64:`MOVD\s\(R.*\)\(R.*<<3\)`,`JMP\s\(R.*\)$`
|
|
switch x {
|
|
case 1:
|
|
return 1
|
|
case 2:
|
|
return 4
|
|
case 3:
|
|
return 9
|
|
case 4:
|
|
return 16
|
|
case 5:
|
|
return 25
|
|
case 6:
|
|
return 36
|
|
case 7:
|
|
return 49
|
|
case 8:
|
|
return 64
|
|
default:
|
|
return x * x
|
|
}
|
|
}
|
|
|
|
// use jump tables for 8+ string lengths
|
|
func length(x string) int {
|
|
// amd64:`JMP\s\(.*\)\(.*\)$`
|
|
// arm64:`MOVD\s\(R.*\)\(R.*<<3\)`,`JMP\s\(R.*\)$`
|
|
switch x {
|
|
case "a":
|
|
return 1
|
|
case "bb":
|
|
return 2
|
|
case "ccc":
|
|
return 3
|
|
case "dddd":
|
|
return 4
|
|
case "eeeee":
|
|
return 5
|
|
case "ffffff":
|
|
return 6
|
|
case "ggggggg":
|
|
return 7
|
|
case "hhhhhhhh":
|
|
return 8
|
|
default:
|
|
return len(x)
|
|
}
|
|
}
|