mirror of
https://github.com/golang/go
synced 2024-09-15 22:20:06 +00:00
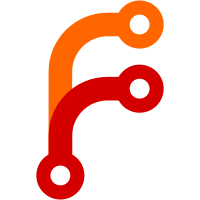
Currently there is a an ANDconst and an ANDCCconst op in PPC64, which is confusing since they map onto the same instruction. One of these ops sets the result of the AND operation, and the other sets the flag (condition register). This converts ANDCCconst into an op with the 2 expected results: the integer result of the AND and the flag setting. The ANDconst op has been removed. Note that in the PPC64 ISA the only variation of the 'and immediate' is the one that sets the condition bit, which probably led to the original (confusing) implementation. This also adds a few rules to improve the use of ANDCCconst with ISELB and some testcases to verify those improvements. Change-Id: I523703fa4da2098eb995dc3ba744d36fa28e41d4 Reviewed-on: https://go-review.googlesource.com/c/go/+/422015 Reviewed-by: Cherry Mui <cherryyz@google.com> Reviewed-by: David Chase <drchase@google.com> Reviewed-by: Paul Murphy <murp@ibm.com>
74 lines
1.9 KiB
Go
74 lines
1.9 KiB
Go
// asmcheck
|
|
|
|
// Copyright 2020 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package codegen
|
|
|
|
// This file contains codegen tests related to boolean simplifications/optimizations.
|
|
|
|
func convertNeq0B(x uint8, c bool) bool {
|
|
// amd64:"ANDL\t[$]1",-"SETNE"
|
|
// ppc64:"ANDCC",-"CMPW",-"ISEL"
|
|
// ppc64le:"ANDCC",-"CMPW",-"ISEL"
|
|
// ppc64le/power9:"ANDCC",-"CMPW",-"ISEL"
|
|
b := x&1 != 0
|
|
return c && b
|
|
}
|
|
|
|
func convertNeq0W(x uint16, c bool) bool {
|
|
// amd64:"ANDL\t[$]1",-"SETNE"
|
|
// ppc64:"ANDCC",-"CMPW",-"ISEL"
|
|
// ppc64le:"ANDCC",-"CMPW",-"ISEL"
|
|
// ppc64le/power9:"ANDCC",-CMPW",-"ISEL"
|
|
b := x&1 != 0
|
|
return c && b
|
|
}
|
|
|
|
func convertNeq0L(x uint32, c bool) bool {
|
|
// amd64:"ANDL\t[$]1",-"SETB"
|
|
// ppc64:"ANDCC",-"CMPW",-"ISEL"
|
|
// ppc64le:"ANDCC",-"CMPW",-"ISEL"
|
|
// ppc64le/power9:"ANDCC",-"CMPW",-"ISEL"
|
|
b := x&1 != 0
|
|
return c && b
|
|
}
|
|
|
|
func convertNeq0Q(x uint64, c bool) bool {
|
|
// amd64:"ANDL\t[$]1",-"SETB"
|
|
// ppc64:"ANDCC",-"CMP",-"ISEL"
|
|
// ppc64le:"ANDCC",-"CMP",-"ISEL"
|
|
// ppc64le/power9:"ANDCC",-"CMP",-"ISEL"
|
|
b := x&1 != 0
|
|
return c && b
|
|
}
|
|
|
|
func convertNeqBool32(x uint32) bool {
|
|
// ppc64:"ANDCC",-"CMPW",-"ISEL"
|
|
// ppc64le:"ANDCC",-"CMPW",-"ISEL"
|
|
// ppc64le/power9:"ANDCC",-"CMPW",-"ISEL"
|
|
return x&1 != 0
|
|
}
|
|
|
|
func convertEqBool32(x uint32) bool {
|
|
// ppc64:"ANDCC",-"CMPW","XOR",-"ISEL"
|
|
// ppc64le:"ANDCC",-"CMPW","XOR",-"ISEL"
|
|
// ppc64le/power9:"ANDCC","XOR",-"CMPW",-"ISEL"
|
|
return x&1 == 0
|
|
}
|
|
|
|
func convertNeqBool64(x uint64) bool {
|
|
// ppc64:"ANDCC",-"CMP",-"ISEL"
|
|
// ppc64le:"ANDCC",-"CMP",-"ISEL"
|
|
// ppc64le/power9:"ANDCC",-"CMP",-"ISEL"
|
|
return x&1 != 0
|
|
}
|
|
|
|
func convertEqBool64(x uint64) bool {
|
|
// ppc64:"ANDCC","XOR",-"CMP",-"ISEL"
|
|
// ppc64le:"ANDCC","XOR",-"CMP",-"ISEL"
|
|
// ppc64le/power9:"ANDCC","XOR",-"CMP",-"ISEL"
|
|
return x&1 == 0
|
|
}
|