mirror of
https://github.com/golang/go
synced 2024-11-02 11:50:30 +00:00
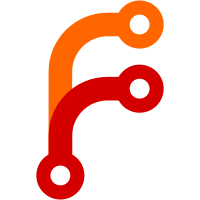
This CL extends unified IR to handle creating wrapper methods. There's relatively little about this code that's actually specific to unified IR, but rewriting this logic allows a few benefits: 1. It decouples unified IR from reflectdata.methodWrapper, so the latter code can evolve freely for -G=3's needs. This will also allow the new code to evolve to unified IR's wrapper needs, which I anticipate will operate slightly differently. 2. It provided an opportunity to revisit a lot of the code and simplify/update it to current style. E.g., in the process, I discovered #46903, which unified IR now gets correctly. (I have not yet attempted to fix reflectdata.methodWrapper.) 3. It gives a convenient way for unified IR to ensure all of the wrapper methods it needs are generated correctly. For now, the wrapper generation is specific to non-quirks mode. Change-Id: I5798de6b141f29e8eb6a5c563e7049627ff2868a Reviewed-on: https://go-review.googlesource.com/c/go/+/330569 Trust: Matthew Dempsky <mdempsky@google.com> Run-TryBot: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Cuong Manh Le <cuong.manhle.vn@gmail.com>
148 lines
1.9 KiB
Go
148 lines
1.9 KiB
Go
// run -gcflags=-G=3
|
|
|
|
// Copyright 2021 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// derived & expanded from cmd/compile/internal/types2/testdata/fixedbugs/issue44688.go2
|
|
|
|
package main
|
|
|
|
type A1[T any] struct{
|
|
val T
|
|
}
|
|
|
|
func (p *A1[T]) m1(val T) {
|
|
p.val = val
|
|
}
|
|
|
|
type A2[T any] interface {
|
|
m2(T)
|
|
}
|
|
|
|
type B1[T any] struct {
|
|
filler int
|
|
*A1[T]
|
|
A2[T]
|
|
}
|
|
|
|
type B2[T any] interface {
|
|
A2[T]
|
|
}
|
|
|
|
type ImpA2[T any] struct {
|
|
f T
|
|
}
|
|
|
|
func (a2 *ImpA2[T]) m2(s T) {
|
|
a2.f = s
|
|
}
|
|
|
|
type C[T any] struct {
|
|
filler1 int
|
|
filler2 int
|
|
B1[T]
|
|
}
|
|
|
|
type D[T any] struct {
|
|
filler1 int
|
|
filler2 int
|
|
filler3 int
|
|
C[T]
|
|
}
|
|
|
|
func test1[T any](arg T) {
|
|
// calling embedded methods
|
|
var b1 B1[T]
|
|
b1.A1 = &A1[T]{}
|
|
b1.A2 = &ImpA2[T]{}
|
|
|
|
b1.A1.m1(arg)
|
|
b1.m1(arg)
|
|
|
|
b1.A2.m2(arg)
|
|
b1.m2(arg)
|
|
|
|
var b2 B2[T]
|
|
b2 = &ImpA2[T]{}
|
|
b2.m2(arg)
|
|
|
|
// a deeper nesting
|
|
var d D[T]
|
|
d.C.B1.A1 = &A1[T]{}
|
|
d.C.B1.A2 = &ImpA2[T]{}
|
|
d.m1(arg)
|
|
d.m2(arg)
|
|
|
|
// calling method expressions
|
|
m1x := B1[T].m1
|
|
m1x(b1, arg)
|
|
m2x := B2[T].m2
|
|
m2x(b2, arg)
|
|
|
|
// calling method values
|
|
m1v := b1.m1
|
|
m1v(arg)
|
|
m2v := b1.m2
|
|
m2v(arg)
|
|
b2v := b2.m2
|
|
b2v(arg)
|
|
}
|
|
|
|
func test2() {
|
|
// calling embedded methods
|
|
var b1 B1[string]
|
|
b1.A1 = &A1[string]{}
|
|
b1.A2 = &ImpA2[string]{}
|
|
|
|
b1.A1.m1("")
|
|
b1.m1("")
|
|
|
|
b1.A2.m2("")
|
|
b1.m2("")
|
|
|
|
var b2 B2[string]
|
|
b2 = &ImpA2[string]{}
|
|
b2.m2("")
|
|
|
|
// a deeper nesting
|
|
var d D[string]
|
|
d.C.B1.A1 = &A1[string]{}
|
|
d.C.B1.A2 = &ImpA2[string]{}
|
|
d.m1("")
|
|
d.m2("")
|
|
|
|
// calling method expressions
|
|
m1x := B1[string].m1
|
|
m1x(b1, "")
|
|
m2x := B2[string].m2
|
|
m2x(b2, "")
|
|
|
|
// calling method values
|
|
m1v := b1.m1
|
|
m1v("")
|
|
m2v := b1.m2
|
|
m2v("")
|
|
b2v := b2.m2
|
|
b2v("")
|
|
}
|
|
|
|
// actual test case from issue
|
|
|
|
type A[T any] struct{}
|
|
|
|
func (*A[T]) f(T) {}
|
|
|
|
type B[T any] struct{ A[T] }
|
|
|
|
func test3() {
|
|
var b B[string]
|
|
b.A.f("")
|
|
b.f("")
|
|
}
|
|
|
|
func main() {
|
|
test1[string]("")
|
|
test2()
|
|
test3()
|
|
}
|