mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
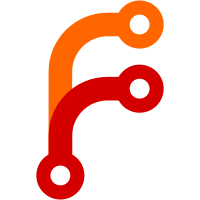
With this change, the shift checking code matches the corresponding go/types code, but for the differences in the internal error reporting, and call of check.overflow. The change leads to the recording of an untyped int value if the RHS of a non-constant shift is an untyped integer value. Adjust the type in the compiler's irgen accordingly. Add test/shift3.go to verify behavior. Change-Id: I20386fcb1d5c48becffdc2203081fb70c08b282d Reviewed-on: https://go-review.googlesource.com/c/go/+/398236 Trust: Robert Griesemer <gri@golang.org> Run-TryBot: Robert Griesemer <gri@golang.org> Trust: Matthew Dempsky <mdempsky@google.com> Reviewed-by: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Gopher Robot <gobot@golang.org> Reviewed-by: Robert Findley <rfindley@google.com>
41 lines
834 B
Go
41 lines
834 B
Go
// run
|
|
|
|
// Copyright 2022 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test that the compiler's noder uses the correct type
|
|
// for RHS shift operands that are untyped. Must compile;
|
|
// run for good measure.
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"math"
|
|
)
|
|
|
|
func f(x, y int) {
|
|
if x != y {
|
|
panic(fmt.Sprintf("%d != %d", x, y))
|
|
}
|
|
}
|
|
|
|
func main() {
|
|
var x int = 1
|
|
f(x<<1, 2)
|
|
f(x<<1., 2)
|
|
f(x<<(1+0i), 2)
|
|
f(x<<0i, 1)
|
|
|
|
f(x<<(1<<x), 4)
|
|
f(x<<(1.<<x), 4)
|
|
f(x<<((1+0i)<<x), 4)
|
|
f(x<<(0i<<x), 1)
|
|
|
|
// corner cases
|
|
const M = math.MaxUint
|
|
f(x<<(M+0), 0) // shift by untyped int representable as uint
|
|
f(x<<(M+0.), 0) // shift by untyped float representable as uint
|
|
f(x<<(M+0.+0i), 0) // shift by untyped complex representable as uint
|
|
}
|