mirror of
https://github.com/golang/go
synced 2024-09-04 23:44:16 +00:00
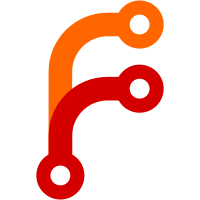
This information is redundant with the position information already provided. Also, no other -m diagnostics print out function name. While here, report parameter leak diagnostics against the parameter declaration position rather than the function, and use Warnl for "moved to heap" messages. Test cases updated programmatically by removing the first word from every "no match for" error emitted by run.go: go run run.go |& \ sed -E -n 's/^(.*):(.*): no match for `([^ ]* (.*))` in:$/\1!\2!\3!\4/p' | \ while IFS='!' read -r fn line before after; do before=$(echo "$before" | sed 's/[.[\*^$()+?{|]/\\&/g') after=$(echo "$after" | sed -E 's/(\&|\\)/\\&/g') fn=$(find . -name "${fn}" | head -1) sed -i -E -e "${line}s/\"${before}\"/\"${after}\"/" "${fn}" done Passes toolstash-check. Change-Id: I6e02486b1409e4a8dbb2b9b816d22095835426b5 Reviewed-on: https://go-review.googlesource.com/c/go/+/195040 Run-TryBot: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Cherry Zhang <cherryyz@google.com>
261 lines
4.5 KiB
Go
261 lines
4.5 KiB
Go
// errorcheck -0 -m -l
|
|
|
|
// Copyright 2015 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test escape analysis for interface conversions.
|
|
|
|
package escape
|
|
|
|
var sink interface{}
|
|
|
|
type M interface {
|
|
M()
|
|
}
|
|
|
|
func mescapes(m M) { // ERROR "leaking param: m"
|
|
sink = m
|
|
}
|
|
|
|
func mdoesnotescape(m M) { // ERROR "m does not escape"
|
|
}
|
|
|
|
// Tests for type stored directly in iface and with value receiver method.
|
|
type M0 struct {
|
|
p *int
|
|
}
|
|
|
|
func (M0) M() {
|
|
}
|
|
|
|
func efaceEscape0() {
|
|
{
|
|
i := 0
|
|
v := M0{&i}
|
|
var x M = v
|
|
_ = x
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := M0{&i}
|
|
var x M = v
|
|
sink = x
|
|
}
|
|
{
|
|
i := 0
|
|
v := M0{&i}
|
|
var x M = v
|
|
v1 := x.(M0)
|
|
_ = v1
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := M0{&i}
|
|
// BAD: v does not escape to heap here
|
|
var x M = v
|
|
v1 := x.(M0)
|
|
sink = v1
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := M0{&i}
|
|
// BAD: v does not escape to heap here
|
|
var x M = v
|
|
x.M()
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := M0{&i}
|
|
var x M = v
|
|
mescapes(x)
|
|
}
|
|
{
|
|
i := 0
|
|
v := M0{&i}
|
|
var x M = v
|
|
mdoesnotescape(x)
|
|
}
|
|
}
|
|
|
|
// Tests for type stored indirectly in iface and with value receiver method.
|
|
type M1 struct {
|
|
p *int
|
|
x int
|
|
}
|
|
|
|
func (M1) M() {
|
|
}
|
|
|
|
func efaceEscape1() {
|
|
{
|
|
i := 0
|
|
v := M1{&i, 0}
|
|
var x M = v // ERROR "v does not escape"
|
|
_ = x
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := M1{&i, 0}
|
|
var x M = v // ERROR "v escapes to heap"
|
|
sink = x
|
|
}
|
|
{
|
|
i := 0
|
|
v := M1{&i, 0}
|
|
var x M = v // ERROR "v does not escape"
|
|
v1 := x.(M1)
|
|
_ = v1
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := M1{&i, 0}
|
|
var x M = v // ERROR "v does not escape"
|
|
v1 := x.(M1)
|
|
sink = v1 // ERROR "v1 escapes to heap"
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := M1{&i, 0}
|
|
// BAD: v does not escape to heap here
|
|
var x M = v // ERROR "v escapes to heap"
|
|
x.M()
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := M1{&i, 0}
|
|
var x M = v // ERROR "v escapes to heap"
|
|
mescapes(x)
|
|
}
|
|
{
|
|
i := 0
|
|
v := M1{&i, 0}
|
|
var x M = v // ERROR "v does not escape"
|
|
mdoesnotescape(x)
|
|
}
|
|
}
|
|
|
|
// Tests for type stored directly in iface and with pointer receiver method.
|
|
type M2 struct {
|
|
p *int
|
|
}
|
|
|
|
func (*M2) M() {
|
|
}
|
|
|
|
func efaceEscape2() {
|
|
{
|
|
i := 0
|
|
v := &M2{&i} // ERROR "&M2 literal does not escape"
|
|
var x M = v
|
|
_ = x
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := &M2{&i} // ERROR "&M2 literal escapes to heap"
|
|
var x M = v
|
|
sink = x
|
|
}
|
|
{
|
|
i := 0
|
|
v := &M2{&i} // ERROR "&M2 literal does not escape"
|
|
var x M = v
|
|
v1 := x.(*M2)
|
|
_ = v1
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := &M2{&i} // ERROR "&M2 literal escapes to heap"
|
|
// BAD: v does not escape to heap here
|
|
var x M = v
|
|
v1 := x.(*M2)
|
|
sink = v1
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := &M2{&i} // ERROR "&M2 literal does not escape"
|
|
// BAD: v does not escape to heap here
|
|
var x M = v
|
|
v1 := x.(*M2)
|
|
sink = *v1
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := &M2{&i} // ERROR "&M2 literal does not escape"
|
|
// BAD: v does not escape to heap here
|
|
var x M = v
|
|
v1, ok := x.(*M2)
|
|
sink = *v1
|
|
_ = ok
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := &M2{&i} // ERROR "&M2 literal escapes to heap"
|
|
// BAD: v does not escape to heap here
|
|
var x M = v
|
|
x.M()
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
v := &M2{&i} // ERROR "&M2 literal escapes to heap"
|
|
var x M = v
|
|
mescapes(x)
|
|
}
|
|
{
|
|
i := 0
|
|
v := &M2{&i} // ERROR "&M2 literal does not escape"
|
|
var x M = v
|
|
mdoesnotescape(x)
|
|
}
|
|
}
|
|
|
|
type T1 struct {
|
|
p *int
|
|
}
|
|
|
|
type T2 struct {
|
|
T1 T1
|
|
}
|
|
|
|
func dotTypeEscape() *T2 { // #11931
|
|
var x interface{}
|
|
x = &T1{p: new(int)} // ERROR "new\(int\) escapes to heap" "&T1 literal does not escape"
|
|
return &T2{
|
|
T1: *(x.(*T1)), // ERROR "&T2 literal escapes to heap"
|
|
}
|
|
}
|
|
|
|
func dotTypeEscape2() { // #13805, #15796
|
|
{
|
|
i := 0
|
|
j := 0
|
|
var v int
|
|
var ok bool
|
|
var x interface{} = i // ERROR "i does not escape"
|
|
var y interface{} = j // ERROR "j does not escape"
|
|
|
|
*(&v) = x.(int)
|
|
*(&v), *(&ok) = y.(int)
|
|
}
|
|
{
|
|
i := 0
|
|
j := 0
|
|
var ok bool
|
|
var x interface{} = i // ERROR "i does not escape"
|
|
var y interface{} = j // ERROR "j does not escape"
|
|
|
|
sink = x.(int) // ERROR "x.\(int\) escapes to heap"
|
|
sink, *(&ok) = y.(int)
|
|
}
|
|
{
|
|
i := 0 // ERROR "moved to heap: i"
|
|
j := 0 // ERROR "moved to heap: j"
|
|
var ok bool
|
|
var x interface{} = &i
|
|
var y interface{} = &j
|
|
|
|
sink = x.(*int)
|
|
sink, *(&ok) = y.(*int)
|
|
}
|
|
}
|