mirror of
https://github.com/golang/go
synced 2024-09-06 00:19:45 +00:00
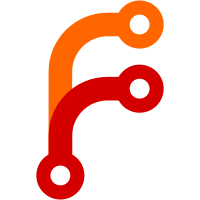
The tests in doc/progs appear to have been originally written for use with the old test driver. At some later point, they acquired their own test driver. Both ran tests in serial. This CL rewrites the current test driver in Go, runs tests concurrently, and cleans up historical artifacts from the old drivers. The primary motivation is to speed up all.bash. On my laptop, using tip, this CL reduces doc/progs test wall time from 26s to 7s. The savings will remain even when the compiler gets faster. Using Go 1.4, this CL reduces test wall time from 15s to 4s. Change-Id: Iae945a8490222beee76e8a2118a0d7956092f543 Reviewed-on: https://go-review.googlesource.com/8410 Reviewed-by: Brad Fitzpatrick <bradfitz@golang.org> Run-TryBot: Josh Bleecher Snyder <josharian@gmail.com> TryBot-Result: Gobot Gobot <gobot@golang.org>
65 lines
860 B
Go
65 lines
860 B
Go
// Copyright 2011 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// This file contains the code snippets included in "Defer, Panic, and Recover."
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"io"
|
|
"os"
|
|
)
|
|
|
|
func a() {
|
|
i := 0
|
|
defer fmt.Println(i)
|
|
i++
|
|
return
|
|
}
|
|
|
|
// STOP OMIT
|
|
|
|
func b() {
|
|
for i := 0; i < 4; i++ {
|
|
defer fmt.Print(i)
|
|
}
|
|
}
|
|
|
|
// STOP OMIT
|
|
|
|
func c() (i int) {
|
|
defer func() { i++ }()
|
|
return 1
|
|
}
|
|
|
|
// STOP OMIT
|
|
|
|
// Initial version.
|
|
func CopyFile(dstName, srcName string) (written int64, err error) {
|
|
src, err := os.Open(srcName)
|
|
if err != nil {
|
|
return
|
|
}
|
|
|
|
dst, err := os.Create(dstName)
|
|
if err != nil {
|
|
return
|
|
}
|
|
|
|
written, err = io.Copy(dst, src)
|
|
dst.Close()
|
|
src.Close()
|
|
return
|
|
}
|
|
|
|
// STOP OMIT
|
|
|
|
func main() {
|
|
a()
|
|
b()
|
|
fmt.Println()
|
|
fmt.Println(c())
|
|
}
|