mirror of
https://github.com/golang/go
synced 2024-07-20 17:48:31 +00:00
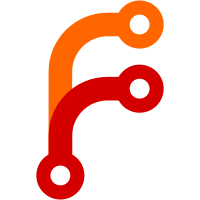
This reverts CL 207477, restoring CL 207352 with a fix for the regression observed in the Windows builders. cmd/compile evidently does not fully support NUL as an output on Windows, so this time we write ignored 'compile' outputs to temporary files (instead of os.DevNull as in CL 207352). Updates #28387 Fixes #35619 Change-Id: I2edc5727c3738fa1bccb4b74e50d114cf2a7fcff Reviewed-on: https://go-review.googlesource.com/c/go/+/207602 Run-TryBot: Bryan C. Mills <bcmills@google.com> Reviewed-by: Brad Fitzpatrick <bradfitz@golang.org> TryBot-Result: Gobot Gobot <gobot@golang.org>
77 lines
1.7 KiB
Go
77 lines
1.7 KiB
Go
// +build !nacl,!js
|
|
// run
|
|
|
|
// Copyright 2014 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Run the sinit test.
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"io/ioutil"
|
|
"os"
|
|
"os/exec"
|
|
"path/filepath"
|
|
"strings"
|
|
)
|
|
|
|
var tmpDir string
|
|
|
|
func cleanup() {
|
|
os.RemoveAll(tmpDir)
|
|
}
|
|
|
|
func run(cmdline ...string) {
|
|
args := strings.Fields(strings.Join(cmdline, " "))
|
|
cmd := exec.Command(args[0], args[1:]...)
|
|
out, err := cmd.CombinedOutput()
|
|
if err != nil {
|
|
fmt.Printf("$ %s\n", cmdline)
|
|
fmt.Println(string(out))
|
|
fmt.Println(err)
|
|
cleanup()
|
|
os.Exit(1)
|
|
}
|
|
}
|
|
|
|
func runFail(cmdline ...string) {
|
|
args := strings.Fields(strings.Join(cmdline, " "))
|
|
cmd := exec.Command(args[0], args[1:]...)
|
|
out, err := cmd.CombinedOutput()
|
|
if err == nil {
|
|
fmt.Printf("$ %s\n", cmdline)
|
|
fmt.Println(string(out))
|
|
fmt.Println("SHOULD HAVE FAILED!")
|
|
cleanup()
|
|
os.Exit(1)
|
|
}
|
|
}
|
|
|
|
func main() {
|
|
var err error
|
|
tmpDir, err = ioutil.TempDir("", "")
|
|
if err != nil {
|
|
fmt.Println(err)
|
|
os.Exit(1)
|
|
}
|
|
tmp := func(name string) string {
|
|
return filepath.Join(tmpDir, name)
|
|
}
|
|
|
|
// helloworld.go is package main
|
|
run("go tool compile -o", tmp("linkmain.o"), "helloworld.go")
|
|
run("go tool compile -pack -o", tmp("linkmain.a"), "helloworld.go")
|
|
run("go tool link -o", tmp("linkmain.exe"), tmp("linkmain.o"))
|
|
run("go tool link -o", tmp("linkmain.exe"), tmp("linkmain.a"))
|
|
|
|
// linkmain.go is not
|
|
run("go tool compile -o", tmp("linkmain1.o"), "linkmain.go")
|
|
run("go tool compile -pack -o", tmp("linkmain1.a"), "linkmain.go")
|
|
runFail("go tool link -o", tmp("linkmain.exe"), tmp("linkmain1.o"))
|
|
runFail("go tool link -o", tmp("linkmain.exe"), tmp("linkmain1.a"))
|
|
cleanup()
|
|
}
|