mirror of
https://github.com/golang/go
synced 2024-07-20 17:48:31 +00:00
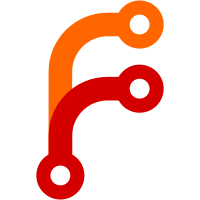
//go:notinheap type T int type U T We already correctly propagate the notinheap-ness of T to U. But we have an assertion in the typechecker that if there's no explicit //go:notinheap associated with U, then report an error. Get rid of that error so that implicit propagation is allowed. Adjust the tests so that we make sure that uses of types like U do correctly report an error when U is used in a context that might cause a Go heap allocation. Fixes #41451 Update #40954 Update #41432 Change-Id: I1692bc7cceff21ebb3f557f3748812a40887118d Reviewed-on: https://go-review.googlesource.com/c/go/+/255637 Run-TryBot: Keith Randall <khr@golang.org> Reviewed-by: Cuong Manh Le <cuong.manhle.vn@gmail.com> Reviewed-by: Ian Lance Taylor <iant@golang.org> Trust: Cuong Manh Le <cuong.manhle.vn@gmail.com> TryBot-Result: Go Bot <gobot@golang.org>
52 lines
1.3 KiB
Go
52 lines
1.3 KiB
Go
// errorcheck -+
|
|
|
|
// Copyright 2016 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test type-checking errors for go:notinheap.
|
|
|
|
package p
|
|
|
|
//go:notinheap
|
|
type nih struct{}
|
|
|
|
type embed4 map[nih]int // ERROR "incomplete \(or unallocatable\) map key not allowed"
|
|
|
|
type embed5 map[int]nih // ERROR "incomplete \(or unallocatable\) map value not allowed"
|
|
|
|
type emebd6 chan nih // ERROR "chan of incomplete \(or unallocatable\) type not allowed"
|
|
|
|
type okay1 *nih
|
|
|
|
type okay2 []nih
|
|
|
|
type okay3 func(x nih) nih
|
|
|
|
type okay4 interface {
|
|
f(x nih) nih
|
|
}
|
|
|
|
// Type conversions don't let you sneak past notinheap.
|
|
|
|
type t1 struct{ x int }
|
|
|
|
//go:notinheap
|
|
type t2 t1
|
|
|
|
//go:notinheap
|
|
type t3 byte
|
|
|
|
//go:notinheap
|
|
type t4 rune
|
|
|
|
var sink interface{}
|
|
|
|
func i() {
|
|
sink = new(t1) // no error
|
|
sink = (*t2)(new(t1)) // ERROR "cannot convert(.|\n)*t2 is incomplete \(or unallocatable\)"
|
|
sink = (*t2)(new(struct{ x int })) // ERROR "cannot convert(.|\n)*t2 is incomplete \(or unallocatable\)"
|
|
sink = []t3("foo") // ERROR "cannot convert(.|\n)*t3 is incomplete \(or unallocatable\)"
|
|
sink = []t4("bar") // ERROR "cannot convert(.|\n)*t4 is incomplete \(or unallocatable\)"
|
|
}
|