mirror of
https://github.com/golang/go
synced 2024-09-06 00:19:45 +00:00
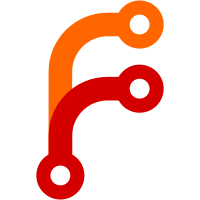
When converting from a generic function to a concrete implementation, add a dictionary argument to the generic function (both an actual argument at each callsite, and a formal argument of each implementation). The dictionary argument comes before all other arguments (including any receiver). The dictionary argument is checked for validity, but is otherwise unused. Subsequent CLs will start using the dictionary for, e.g., converting a value of generic type to interface{}. Import/export required adding support for LINKSYMOFFSET, which is used by the dictionary checking code. Change-Id: I16a7a8d23c7bd6a897e0da87c69f273be9103bd7 Reviewed-on: https://go-review.googlesource.com/c/go/+/323272 Trust: Keith Randall <khr@golang.org> Trust: Dan Scales <danscales@google.com> Run-TryBot: Keith Randall <khr@golang.org> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Dan Scales <danscales@google.com>
101 lines
1.5 KiB
Go
101 lines
1.5 KiB
Go
// run -gcflags=-G=3
|
|
|
|
// Copyright 2021 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test situations where functions/methods are not
|
|
// immediately called and we need to capture the dictionary
|
|
// required for later invocation.
|
|
|
|
// TODO: copy this test file, add -l to gcflags.
|
|
|
|
package main
|
|
|
|
func main() {
|
|
functions()
|
|
methodExpressions()
|
|
methodValues()
|
|
interfaceMethods()
|
|
}
|
|
|
|
func g0[T any](x T) {
|
|
}
|
|
func g1[T any](x T) T {
|
|
return x
|
|
}
|
|
func g2[T any](x T) (T, T) {
|
|
return x, x
|
|
}
|
|
|
|
func functions() {
|
|
f0 := g0[int]
|
|
f0(7)
|
|
f1 := g1[int]
|
|
is7(f1(7))
|
|
f2 := g2[int]
|
|
is77(f2(7))
|
|
}
|
|
|
|
func is7(x int) {
|
|
if x != 7 {
|
|
println(x)
|
|
panic("assertion failed")
|
|
}
|
|
}
|
|
func is77(x, y int) {
|
|
if x != 7 || y != 7 {
|
|
println(x,y)
|
|
panic("assertion failed")
|
|
}
|
|
}
|
|
|
|
type s[T any] struct {
|
|
a T
|
|
}
|
|
|
|
func (x s[T]) g0() {
|
|
}
|
|
func (x s[T]) g1() T {
|
|
return x.a
|
|
}
|
|
func (x s[T]) g2() (T, T) {
|
|
return x.a, x.a
|
|
}
|
|
|
|
func methodExpressions() {
|
|
x := s[int]{a:7}
|
|
f0 := s[int].g0
|
|
f0(x)
|
|
f1 := s[int].g1
|
|
is7(f1(x))
|
|
f2 := s[int].g2
|
|
is77(f2(x))
|
|
}
|
|
|
|
func methodValues() {
|
|
x := s[int]{a:7}
|
|
f0 := x.g0
|
|
f0()
|
|
f1 := x.g1
|
|
is7(f1())
|
|
f2 := x.g2
|
|
is77(f2())
|
|
}
|
|
|
|
var x interface{
|
|
g0()
|
|
g1()int
|
|
g2()(int,int)
|
|
} = s[int]{a:7}
|
|
var y interface{} = s[int]{a:7}
|
|
|
|
func interfaceMethods() {
|
|
x.g0()
|
|
is7(x.g1())
|
|
is77(x.g2())
|
|
y.(interface{g0()}).g0()
|
|
is7(y.(interface{g1()int}).g1())
|
|
is77(y.(interface{g2()(int,int)}).g2())
|
|
}
|