mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
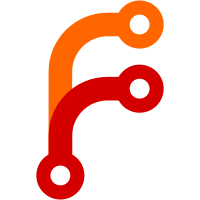
unsafe.SliceData can return pointers which are nil. That function gets lowered to the SSA OpSlicePtr, which the compiler assumes is non-nil. This used to be the case as OpSlicePtr was only used in situations where the bounds check already passed. But with unsafe.SliceData that is no longer the case. There are situations where we know it is nil. Use Bounded() to indicate that. I looked through all the uses of OSPTR and added SetBounded where it made sense. Most OSPTR results are passed directly to runtime calls (e.g. memmove), so even if we know they are non-nil that info isn't helpful. Fixes #59293 Change-Id: I437a15330db48e0082acfb1f89caf8c56723fc51 Reviewed-on: https://go-review.googlesource.com/c/go/+/479896 Reviewed-by: Matthew Dempsky <mdempsky@google.com> Reviewed-by: Keith Randall <khr@google.com> TryBot-Result: Gopher Robot <gobot@golang.org> Run-TryBot: Keith Randall <khr@golang.org>
29 lines
433 B
Go
29 lines
433 B
Go
// run
|
|
|
|
// Copyright 2023 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import "unsafe"
|
|
|
|
//go:noinline
|
|
func f(x []byte) bool {
|
|
return unsafe.SliceData(x) != nil
|
|
}
|
|
|
|
//go:noinline
|
|
func g(x string) bool {
|
|
return unsafe.StringData(x) != nil
|
|
}
|
|
|
|
func main() {
|
|
if f(nil) {
|
|
panic("bad f")
|
|
}
|
|
if g("") {
|
|
panic("bad g")
|
|
}
|
|
}
|