mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
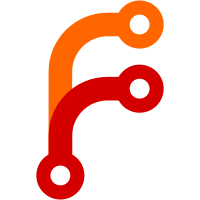
In the Sizes API, recognize an overflow (to a negative value) as a consequence of an oversize value, and specify as such in the API. Adjust the various size computations to take overflow into account. Recognize a negative size or offset as an error and report it rather than panicking. Use the same protocol for results provided by the default (StdSizes) and external Sizes implementations. Add a new error code TypeTooLarge for the new errors. Fixes #59190. Fixes #59207. Change-Id: I8c33a9e69932760275100112dde627289ac7695b Reviewed-on: https://go-review.googlesource.com/c/go/+/478919 Run-TryBot: Robert Griesemer <gri@google.com> Reviewed-by: Robert Findley <rfindley@google.com> TryBot-Result: Gopher Robot <gobot@golang.org> Auto-Submit: Robert Griesemer <gri@google.com> Reviewed-by: Robert Griesemer <gri@google.com>
40 lines
688 B
Go
40 lines
688 B
Go
// errorcheck
|
|
|
|
// Copyright 2023 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package p
|
|
|
|
import "unsafe"
|
|
|
|
type E [1 << 30]complex128
|
|
|
|
var a [1 << 30]E
|
|
var _ = unsafe.Sizeof(a) // ERROR "too large"
|
|
|
|
var s struct {
|
|
_ [1 << 30]E
|
|
x int
|
|
}
|
|
var _ = unsafe.Offsetof(s.x) // ERROR "too large"
|
|
|
|
// Test case from issue (modified so it also triggers on 32-bit platforms).
|
|
|
|
type A [1]int
|
|
type S struct {
|
|
x A
|
|
y [1 << 30]A
|
|
z [1 << 30]struct{}
|
|
}
|
|
type T [1 << 30][1 << 30]S
|
|
|
|
func _() {
|
|
var a A
|
|
var s S
|
|
var t T
|
|
_ = unsafe.Sizeof(a)
|
|
_ = unsafe.Sizeof(s)
|
|
_ = unsafe.Sizeof(t) // ERROR "too large"
|
|
}
|