mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
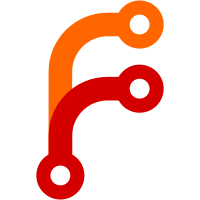
I structured the test for issue54343.go after issue46725.go, where I was careful to use `[4]int`, which is a type large enough to avoid the tiny object allocator (which interferes with finalizer semantics). But in that test, I didn't note the importance of that type, so I mistakenly used just `int` in issue54343.go. This CL switches issue54343.go to use `[4]int` too, and then adds comments to both pointing out the significance of this type. Updates #54343. Change-Id: I699b3e64b844ff6d8438bbcb4d1935615a6d8cc4 Reviewed-on: https://go-review.googlesource.com/c/go/+/423115 TryBot-Result: Gopher Robot <gobot@golang.org> Run-TryBot: Matthew Dempsky <mdempsky@google.com> Reviewed-by: David Chase <drchase@google.com>
46 lines
695 B
Go
46 lines
695 B
Go
// run
|
|
|
|
// Copyright 2022 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import "runtime"
|
|
|
|
func main() {
|
|
if wait() {
|
|
panic("GC'd early")
|
|
}
|
|
m = nil
|
|
if !wait() {
|
|
panic("never GC'd")
|
|
}
|
|
}
|
|
|
|
var m = New[int]().M
|
|
|
|
func New[X any]() *T[X] {
|
|
p := new(T[X])
|
|
runtime.SetFinalizer(p, func(*T[X]) { close(done) })
|
|
return p
|
|
}
|
|
|
|
type T[X any] [4]int // N.B., [4]int avoids runtime's tiny object allocator
|
|
|
|
func (*T[X]) M() {}
|
|
|
|
var done = make(chan int)
|
|
|
|
func wait() bool {
|
|
for i := 0; i < 10; i++ {
|
|
runtime.GC()
|
|
select {
|
|
case <-done:
|
|
return true
|
|
default:
|
|
}
|
|
}
|
|
return false
|
|
}
|