mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
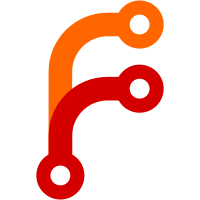
A composite literal assignment x = T{field: v} may be compiled to x = T{} x.field = v We already do not use this form is RHS uses LHS. If LHS is address-taken, RHS may uses LHS implicitly, e.g. v = &x.field x = T{field: *v} The lowering above would change the value of RHS (*v). Fixes #52953. Change-Id: I3f798e00598aaa550b8c17182c7472fef440d483 Reviewed-on: https://go-review.googlesource.com/c/go/+/407014 Reviewed-by: Cuong Manh Le <cuong.manhle.vn@gmail.com> Run-TryBot: Cherry Mui <cherryyz@google.com> TryBot-Result: Gopher Robot <gobot@golang.org> Reviewed-by: Michael Knyszek <mknyszek@google.com>
30 lines
488 B
Go
30 lines
488 B
Go
// run
|
|
|
|
// Copyright 2022 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Issue 52953: miscompilation for composite literal assignment
|
|
// when LHS is address-taken.
|
|
|
|
package main
|
|
|
|
type T struct {
|
|
Field1 bool
|
|
}
|
|
|
|
func main() {
|
|
var ret T
|
|
ret.Field1 = true
|
|
var v *bool = &ret.Field1
|
|
ret = T{Field1: *v}
|
|
check(ret.Field1)
|
|
}
|
|
|
|
//go:noinline
|
|
func check(b bool) {
|
|
if !b {
|
|
panic("FAIL")
|
|
}
|
|
}
|