mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
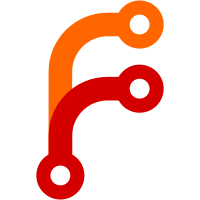
We need to use the same marker everywhere. My CL to rename the marker (CL 241661) and the CL to add more uses of the marker under the old name (CL 241678) weren't coordinated with each other. Fixes #52612 Change-Id: I97023c0769e518491924ef457fe03bf64a2cefa6 Reviewed-on: https://go-review.googlesource.com/c/go/+/403094 Run-TryBot: Keith Randall <khr@golang.org> Reviewed-by: Ian Lance Taylor <iant@google.com> TryBot-Result: Gopher Robot <gobot@golang.org> Reviewed-by: Keith Randall <khr@google.com>
50 lines
881 B
Go
50 lines
881 B
Go
// run
|
|
|
|
// Copyright 2022 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import (
|
|
"sync/atomic"
|
|
"unsafe"
|
|
)
|
|
|
|
var one interface{} = 1
|
|
|
|
type eface struct {
|
|
typ unsafe.Pointer
|
|
data unsafe.Pointer
|
|
}
|
|
|
|
func f(c chan struct{}) {
|
|
var x atomic.Value
|
|
|
|
go func() {
|
|
x.Swap(one) // writing using the old marker
|
|
}()
|
|
for i := 0; i < 100000; i++ {
|
|
v := x.Load() // reading using the new marker
|
|
|
|
p := (*eface)(unsafe.Pointer(&v)).typ
|
|
if uintptr(p) == ^uintptr(0) {
|
|
// We read the old marker, which the new reader
|
|
// doesn't know is a case where it should retry
|
|
// instead of returning it.
|
|
panic("bad typ field")
|
|
}
|
|
}
|
|
c <- struct{}{}
|
|
}
|
|
|
|
func main() {
|
|
c := make(chan struct{}, 10)
|
|
for i := 0; i < 10; i++ {
|
|
go f(c)
|
|
}
|
|
for i := 0; i < 10; i++ {
|
|
<-c
|
|
}
|
|
}
|