mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
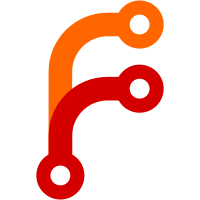
We use AutogeneratedPos for most compiler-generated functions. But for method value wrappers we currently don't. Instead, we use the Pos for their (direct) declaration if there is one, otherwise not set it in methodValueWrapper, which will probably cause it to inherit from the caller, i.e. the Pos of that method value expression. If that Pos has inline information, it will cause the method wrapper to have bogus inline information, which could lead to infinite loop when printing a stack trace. Change it to use AutogeneratedPos instead. Fixes #51401. Change-Id: I398dfe85f9f875e1fd82dc2f489dab63ada6570d Reviewed-on: https://go-review.googlesource.com/c/go/+/388794 Trust: Cherry Mui <cherryyz@google.com> Run-TryBot: Cherry Mui <cherryyz@google.com> TryBot-Result: Gopher Robot <gobot@golang.org> Reviewed-by: Matthew Dempsky <mdempsky@google.com>
45 lines
766 B
Go
45 lines
766 B
Go
// run
|
|
|
|
// Copyright 2022 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Issue 51401: bad inline info in generated interface method wrapper
|
|
// causes infinite loop in stack unwinding.
|
|
|
|
package main
|
|
|
|
import "runtime"
|
|
|
|
type Outer interface{ Inner }
|
|
|
|
type impl struct{}
|
|
|
|
func New() Outer { return &impl{} }
|
|
|
|
type Inner interface {
|
|
DoStuff() error
|
|
}
|
|
|
|
func (a *impl) DoStuff() error {
|
|
return newError()
|
|
}
|
|
|
|
func newError() error {
|
|
stack := make([]uintptr, 50)
|
|
runtime.Callers(2, stack[:])
|
|
|
|
return nil
|
|
}
|
|
|
|
func main() {
|
|
funcs := listFuncs(New())
|
|
for _, f := range funcs {
|
|
f()
|
|
}
|
|
}
|
|
|
|
func listFuncs(outer Outer) []func() error {
|
|
return []func() error{outer.DoStuff}
|
|
}
|