mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
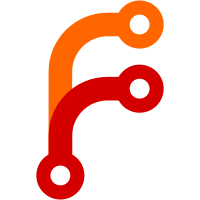
For an extension operation like MOWWreg, if the operand is already extended, we optimize the second extension out. Usually a LoadReg of a proper type would come already extended, as a MOVW/MOVWU etc. instruction does. But for a LoadReg to a floating point register, the instruction does not do the extension. So we cannot elide the extension. Fixes #50671. Change-Id: Id8991df78d5acdecd3fd6138c558428cbd5f6ba3 Reviewed-on: https://go-review.googlesource.com/c/go/+/379236 Trust: Cherry Mui <cherryyz@google.com> Run-TryBot: Cherry Mui <cherryyz@google.com> TryBot-Result: Gopher Robot <gobot@golang.org> Reviewed-by: David Chase <drchase@google.com>
36 lines
599 B
Go
36 lines
599 B
Go
// run
|
|
|
|
// Copyright 2022 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Issue 50671: sign extension eliminated incorrectly on MIPS64.
|
|
|
|
package main
|
|
|
|
//go:noinline
|
|
func F(x int32) (float64, int64) {
|
|
a := float64(x)
|
|
b := int64(x)
|
|
return a, b
|
|
}
|
|
|
|
var a, b, c float64
|
|
|
|
// Poison some floating point registers with non-zero high bits.
|
|
//
|
|
//go:noinline
|
|
func poison(x float64) {
|
|
a = x - 123.45
|
|
b = a * 1.2
|
|
c = b + 3.4
|
|
}
|
|
|
|
func main() {
|
|
poison(333.3)
|
|
_, b := F(123)
|
|
if b != 123 {
|
|
panic("FAIL")
|
|
}
|
|
}
|