mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
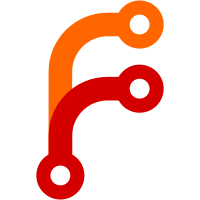
checkptr has code to recognize &^ expressions, but it didn't take into account that "p &^ x" gets rewritten to "p & ^x" during walk, which resulted in false positive diagnostics. This CL changes walkexpr to mark OANDNOT expressions with Implicit when they're rewritten to OAND, so that walkCheckPtrArithmetic can still recognize them later. It would be slightly more idiomatic to instead mark the OBITNOT expression as Implicit (as it's a compiler-generated Node), but the OBITNOT expression might get constant folded. It's not worth the extra complexity/subtlety of relying on n.Right.Orig, so we set Implicit on the OAND node instead. To atone for this transgression, I add documentation for nodeImplicit. Fixes #40917. Change-Id: I386304171ad299c530e151e5924f179e9a5fd5b8 Reviewed-on: https://go-review.googlesource.com/c/go/+/249477 Run-TryBot: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Keith Randall <khr@golang.org> Reviewed-by: Cuong Manh Le <cuong.manhle.vn@gmail.com>
24 lines
453 B
Go
24 lines
453 B
Go
// run -gcflags=-d=checkptr
|
|
|
|
// Copyright 2020 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import "unsafe"
|
|
|
|
func main() {
|
|
var x [2]uint64
|
|
a := unsafe.Pointer(&x[1])
|
|
|
|
b := a
|
|
b = unsafe.Pointer(uintptr(b) + 2)
|
|
b = unsafe.Pointer(uintptr(b) - 1)
|
|
b = unsafe.Pointer(uintptr(b) &^ 1)
|
|
|
|
if a != b {
|
|
panic("pointer arithmetic failed")
|
|
}
|
|
}
|