mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
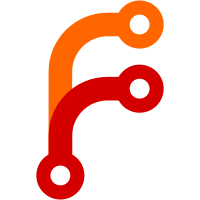
When inserting Select0 and Select1 ops we need to ensure that they live in the same block as their argument. This is because they need to be scheduled immediately after their argument for register and flag allocation to work correctly. Fixes #38356. Change-Id: Iba384dbe87010f1c7c4ce909f08011e5f1de7fd5 Reviewed-on: https://go-review.googlesource.com/c/go/+/227879 Run-TryBot: Michael Munday <mike.munday@ibm.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Keith Randall <khr@golang.org>
55 lines
1.1 KiB
Go
55 lines
1.1 KiB
Go
// compile
|
|
|
|
// Copyright 2020 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Make sure floating point operations that generate flags
|
|
// are scheduled correctly on s390x.
|
|
|
|
package p
|
|
|
|
func f1(x, y float64, z int) float64 {
|
|
a := x + y // generate flags
|
|
if z == 0 { // create basic block that does not clobber flags
|
|
return a
|
|
}
|
|
if a > 0 { // use flags in different basic block
|
|
return y
|
|
}
|
|
return x
|
|
}
|
|
|
|
func f2(x, y float64, z int) float64 {
|
|
a := x - y // generate flags
|
|
if z == 0 { // create basic block that does not clobber flags
|
|
return a
|
|
}
|
|
if a > 0 { // use flags in different basic block
|
|
return y
|
|
}
|
|
return x
|
|
}
|
|
|
|
func f3(x, y float32, z int) float32 {
|
|
a := x + y // generate flags
|
|
if z == 0 { // create basic block that does not clobber flags
|
|
return a
|
|
}
|
|
if a > 0 { // use flags in different basic block
|
|
return y
|
|
}
|
|
return x
|
|
}
|
|
|
|
func f4(x, y float32, z int) float32 {
|
|
a := x - y // generate flags
|
|
if z == 0 { // create basic block that does not clobber flags
|
|
return a
|
|
}
|
|
if a > 0 { // use flags in different basic block
|
|
return y
|
|
}
|
|
return x
|
|
}
|