mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
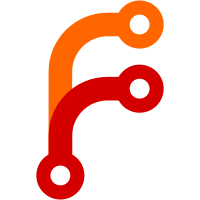
On PPC64, MOVWload, MOVDload, and MOVDstore are assembled to a "DS from" instruction which requiers the offset is a multiple of 4. Only fold offset to such instructions if it is a multiple of 4. Fixes #36723. "GOARCH=ppc64 GOOS=linux go build -gcflags=all=-d=ssa/check/on std cmd" passes now. Change-Id: I67f2a6ac02f0d33d470f68ff54936c289a4c765b Reviewed-on: https://go-review.googlesource.com/c/go/+/216379 Reviewed-by: Carlos Eduardo Seo <cseo@linux.vnet.ibm.com>
27 lines
630 B
Go
27 lines
630 B
Go
// compile -d=ssa/check/on
|
|
|
|
// Copyright 2020 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Issue 36723: fail to compile on PPC64 when SSA check is on.
|
|
|
|
package p
|
|
|
|
import "unsafe"
|
|
|
|
type T struct {
|
|
a, b, c, d uint8
|
|
x [10]int32
|
|
}
|
|
|
|
func F(p *T, i uintptr) int32 {
|
|
// load p.x[i] using unsafe, derived from runtime.pcdatastart
|
|
_ = *p
|
|
return *(*int32)(add(unsafe.Pointer(&p.d), unsafe.Sizeof(p.d)+i*unsafe.Sizeof(p.x[0])))
|
|
}
|
|
|
|
func add(p unsafe.Pointer, x uintptr) unsafe.Pointer {
|
|
return unsafe.Pointer(uintptr(p) + x)
|
|
}
|