mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
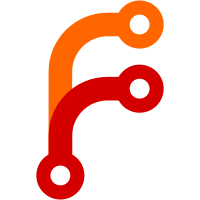
The logic for detecting deferreturn calls is wrong. We used to look for a relocation whose symbol is runtime.deferreturn and has an offset of 0. But on some architectures, the relocation offset is not zero. These include arm (the offset is 0xebfffffe) and s390x (the offset is 6). This ends up setting the deferreturn offset at 0, so we end up using the entry point live map instead of the deferreturn live map in a frame which defers and then segfaults. Instead, use the IsDirectCall helper to find calls. Fixes #32477 Update #6980 Change-Id: Iecb530a7cf6eabd7233be7d0731ffa78873f3a54 Reviewed-on: https://go-review.googlesource.com/c/go/+/181258 Run-TryBot: Keith Randall <khr@golang.org> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Cherry Zhang <cherryyz@google.com>
72 lines
1.2 KiB
Go
72 lines
1.2 KiB
Go
// run
|
|
|
|
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Make sure we use the deferreturn live map instead of
|
|
// the entry live map when handling a segv in a function
|
|
// that defers.
|
|
|
|
package main
|
|
|
|
import "runtime"
|
|
|
|
var finalized bool
|
|
var err string
|
|
|
|
type HeapObj [8]int64
|
|
|
|
const filler int64 = 0x123456789abcdef0
|
|
|
|
func (h *HeapObj) init() {
|
|
for i := 0; i < len(*h); i++ {
|
|
h[i] = filler
|
|
}
|
|
}
|
|
func (h *HeapObj) check() {
|
|
for i := 0; i < len(*h); i++ {
|
|
if h[i] != filler {
|
|
err = "filler overwritten"
|
|
}
|
|
}
|
|
}
|
|
|
|
func gc(shouldFinalize bool) {
|
|
runtime.GC()
|
|
runtime.GC()
|
|
runtime.GC()
|
|
if shouldFinalize != finalized {
|
|
err = "heap object finalized at the wrong time"
|
|
}
|
|
}
|
|
|
|
func main() {
|
|
h := new(HeapObj)
|
|
h.init()
|
|
runtime.SetFinalizer(h, func(h *HeapObj) {
|
|
finalized = true
|
|
})
|
|
|
|
gc(false)
|
|
g(h)
|
|
if err != "" {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
func g(h *HeapObj) {
|
|
gc(false)
|
|
h.check()
|
|
// h is now unused
|
|
defer func() {
|
|
// h should not be live here. Previously we used to
|
|
// use the function entry point as the place to get
|
|
// the live map when handling a segv.
|
|
gc(true)
|
|
recover()
|
|
}()
|
|
*(*int)(nil) = 0 // trigger a segv
|
|
return
|
|
}
|