mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
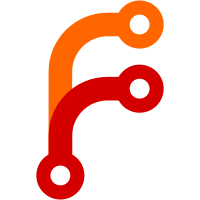
Currently, we rewrite: go f(new(T)) into: tmp := new(T) go func() { f(tmp) }() However, we can both shrink the closure and improve escape analysis by instead rewriting it into: go func() { f(new(T)) }() This CL does that. Change-Id: Iae16a476368da35123052ca9ff41c49159980458 Reviewed-on: https://go-review.googlesource.com/c/go/+/520340 TryBot-Result: Gopher Robot <gobot@golang.org> Reviewed-by: Cuong Manh Le <cuong.manhle.vn@gmail.com> Run-TryBot: Matthew Dempsky <mdempsky@google.com> Auto-Submit: Matthew Dempsky <mdempsky@google.com> Reviewed-by: Dmitri Shuralyov <dmitshur@google.com>
50 lines
2.3 KiB
Go
50 lines
2.3 KiB
Go
// errorcheck -0 -m -l
|
|
|
|
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package p
|
|
|
|
func f(...*int) {}
|
|
|
|
func g() {
|
|
defer f()
|
|
defer f(new(int)) // ERROR "... argument does not escape$" "new\(int\) does not escape$"
|
|
defer f(new(int), new(int)) // ERROR "... argument does not escape$" "new\(int\) does not escape$"
|
|
|
|
defer f(nil...)
|
|
defer f([]*int{}...) // ERROR "\[\]\*int{} does not escape$"
|
|
defer f([]*int{new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) does not escape$"
|
|
defer f([]*int{new(int), new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) does not escape$"
|
|
|
|
go f()
|
|
go f(new(int)) // ERROR "... argument does not escape$" "new\(int\) does not escape$"
|
|
go f(new(int), new(int)) // ERROR "... argument does not escape$" "new\(int\) does not escape$"
|
|
|
|
go f(nil...)
|
|
go f([]*int{}...) // ERROR "\[\]\*int{} does not escape$"
|
|
go f([]*int{new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) does not escape$"
|
|
go f([]*int{new(int), new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) does not escape$"
|
|
|
|
for {
|
|
defer f()
|
|
defer f(new(int)) // ERROR "... argument does not escape$" "new\(int\) does not escape$"
|
|
defer f(new(int), new(int)) // ERROR "... argument does not escape$" "new\(int\) does not escape$"
|
|
|
|
defer f(nil...)
|
|
defer f([]*int{}...) // ERROR "\[\]\*int{} does not escape$"
|
|
defer f([]*int{new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) does not escape$"
|
|
defer f([]*int{new(int), new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) does not escape$"
|
|
|
|
go f()
|
|
go f(new(int)) // ERROR "... argument does not escape$" "new\(int\) does not escape$"
|
|
go f(new(int), new(int)) // ERROR "... argument does not escape$" "new\(int\) does not escape$"
|
|
|
|
go f(nil...)
|
|
go f([]*int{}...) // ERROR "\[\]\*int{} does not escape$"
|
|
go f([]*int{new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) does not escape$"
|
|
go f([]*int{new(int), new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) does not escape$"
|
|
}
|
|
}
|