mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
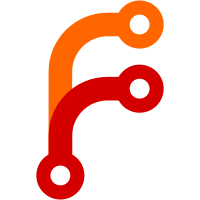
There are several places where a new (internal) complex constant is allocated via new(Mpcplx) rather than newMpcmplx(). The problem with using new() is that the Mpcplx data structure's Real and Imag components don't get initialized with an Mpflt of the correct precision (they have precision 0, which may be adjusted later). In all cases but one, the components of those complex constants are set using a Set operation which "inherits" the correct precision from the value that is being set. But when creating a complex value for an imaginary literal, the imaginary component is set via SetString which assumes 64bits of precision by default. As a result, the internal representation of 0.01i and complex(0, 0.01) was not correct. Replaced all used of new(Mpcplx) with newMpcmplx() and added a new test. Fixes #30243. Change-Id: Ife7fd6ccd42bf887a55c6ce91727754657e6cb2d Reviewed-on: https://go-review.googlesource.com/c/163000 Run-TryBot: Robert Griesemer <gri@golang.org> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Matthew Dempsky <mdempsky@google.com>
28 lines
578 B
Go
28 lines
578 B
Go
// run
|
|
|
|
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Compile-time constants, even if they cannot be represented
|
|
// accurately, should remain the same in operations that don't
|
|
// affect their values.
|
|
|
|
package main
|
|
|
|
import "fmt"
|
|
|
|
func main() {
|
|
const x = 0.01
|
|
const xi = 0.01i
|
|
const xc = complex(0, x)
|
|
|
|
if imag(xi) != x {
|
|
fmt.Printf("FAILED: %g != %g\n", imag(xi), x)
|
|
}
|
|
|
|
if xi != complex(0, x) {
|
|
fmt.Printf("FAILED: %g != %g\n", xi, complex(0, x))
|
|
}
|
|
}
|