mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
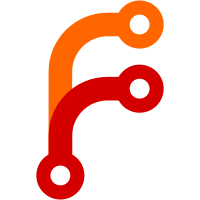
In some optimization rules the type of generated OffPtr was incorrectly set to the type of the pointee, instead of the pointer. When the OffPtr value is spilled, this may generate a spill of the wrong type, e.g. a floating point spill of an integer (pointer) value. On Wasm, this leads to invalid bytecode. Fixes #27961. Change-Id: I5d464847eb900ed90794105c0013a1a7330756cc Reviewed-on: https://go-review.googlesource.com/c/139257 Run-TryBot: Cherry Zhang <cherryyz@google.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Keith Randall <khr@golang.org> Reviewed-by: Richard Musiol <neelance@gmail.com>
36 lines
596 B
Go
36 lines
596 B
Go
// run
|
|
|
|
// Copyright 2018 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Issue 27961: some optimizations generate OffPtr with wrong
|
|
// types, which causes invalid bytecode on Wasm.
|
|
|
|
package main
|
|
|
|
import "math"
|
|
|
|
type Vec2 [2]float64
|
|
|
|
func main() {
|
|
var a Vec2
|
|
a.A().B().C().D()
|
|
}
|
|
|
|
func (v Vec2) A() Vec2 {
|
|
return Vec2{v[0], v[0]}
|
|
}
|
|
|
|
func (v Vec2) B() Vec2 {
|
|
return Vec2{1.0 / v.D(), 0}
|
|
}
|
|
|
|
func (v Vec2) C() Vec2 {
|
|
return Vec2{v[0], v[0]}
|
|
}
|
|
|
|
func (v Vec2) D() float64 {
|
|
return math.Sqrt(v[0])
|
|
}
|