mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
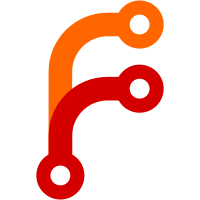
Functions exported on behalf of other packages need to have their argument stack maps specified explicitly. They don't get an implicit map because they are not in the local package, and if they get defer'd they need argument maps. Fixes #24419 Change-Id: I35b7d8b4a03d4770ba88699e1007cb3fcb5397a9 Reviewed-on: https://go-review.googlesource.com/122676 Run-TryBot: Keith Randall <khr@golang.org> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Cherry Zhang <cherryyz@google.com>
52 lines
813 B
Go
52 lines
813 B
Go
// run
|
|
|
|
// Copyright 2018 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import (
|
|
"bytes"
|
|
"strings"
|
|
)
|
|
|
|
func growstack(n int) {
|
|
if n > 0 {
|
|
growstack(n - 1)
|
|
}
|
|
}
|
|
|
|
func main() {
|
|
c := make(chan struct{})
|
|
go compare(c)
|
|
go equal(c)
|
|
go indexByte(c)
|
|
go indexByteString(c)
|
|
<-c
|
|
<-c
|
|
<-c
|
|
<-c
|
|
}
|
|
|
|
func compare(c chan struct{}) {
|
|
defer bytes.Compare(nil, nil)
|
|
growstack(10000)
|
|
c <- struct{}{}
|
|
}
|
|
func equal(c chan struct{}) {
|
|
defer bytes.Equal(nil, nil)
|
|
growstack(10000)
|
|
c <- struct{}{}
|
|
}
|
|
func indexByte(c chan struct{}) {
|
|
defer bytes.IndexByte(nil, 0)
|
|
growstack(10000)
|
|
c <- struct{}{}
|
|
}
|
|
func indexByteString(c chan struct{}) {
|
|
defer strings.IndexByte("", 0)
|
|
growstack(10000)
|
|
c <- struct{}{}
|
|
}
|