mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
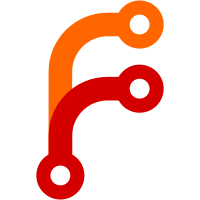
A Select Op could produce a value with upper 32 bits NOT zeroed, for example, Div32 is lowered to (Select0 (DIVL x y)). In theory, we could look into the argument of a Select to decide whether the upper bits are zeroed. As it is late in release cycle, just disable this optimization for Select for now. Fixes #23305. Change-Id: Icf665a2af9ccb0a7ba0ae00c683c9e349638bf85 Reviewed-on: https://go-review.googlesource.com/85736 Run-TryBot: Cherry Zhang <cherryyz@google.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Matthew Dempsky <mdempsky@google.com> Reviewed-by: Ilya Tocar <ilya.tocar@intel.com>
29 lines
575 B
Go
29 lines
575 B
Go
// run
|
|
|
|
// Copyright 2018 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
func mask1(a, b uint64) uint64 {
|
|
op1 := int32(a)
|
|
op2 := int32(b)
|
|
return uint64(uint32(op1 / op2))
|
|
}
|
|
|
|
var mask2 = mask1
|
|
|
|
func main() {
|
|
res1 := mask1(0x1, 0xfffffffeffffffff)
|
|
res2 := mask2(0x1, 0xfffffffeffffffff)
|
|
if res1 != 0xffffffff {
|
|
println("got", res1, "want", 0xffffffff)
|
|
panic("FAIL")
|
|
}
|
|
if res2 != 0xffffffff {
|
|
println("got", res2, "want", 0xffffffff)
|
|
panic("FAIL")
|
|
}
|
|
}
|