mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
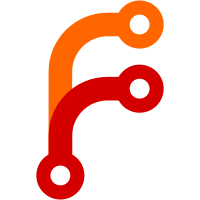
Use entire inlining call stack to decide whether two panic calls can be merged. We used to merge panic calls when only the leaf line numbers matched, but that leads to places higher up the call stack being merged incorrectly. Fixes #22083 Change-Id: Ia41400a80de4b6ecf3e5089abce0c42b65e9b38a Reviewed-on: https://go-review.googlesource.com/67632 Run-TryBot: Emmanuel Odeke <emm.odeke@gmail.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Robert Griesemer <gri@golang.org>
42 lines
791 B
Go
42 lines
791 B
Go
// run
|
|
|
|
// Copyright 2017 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// The compiler was panicking on the wrong line number, where
|
|
// the panic was occurring in an inlined call.
|
|
|
|
package main
|
|
|
|
import (
|
|
"runtime/debug"
|
|
"strings"
|
|
)
|
|
|
|
type Wrapper struct {
|
|
a []int
|
|
}
|
|
|
|
func (w Wrapper) Get(i int) int {
|
|
return w.a[i]
|
|
}
|
|
|
|
func main() {
|
|
defer func() {
|
|
e := recover()
|
|
if e == nil {
|
|
panic("bounds check didn't fail")
|
|
}
|
|
stk := string(debug.Stack())
|
|
if !strings.Contains(stk, "issue22083.go:40") {
|
|
panic("wrong stack trace: " + stk)
|
|
}
|
|
}()
|
|
foo := Wrapper{a: []int{0, 1, 2}}
|
|
_ = foo.Get(0)
|
|
_ = foo.Get(1)
|
|
_ = foo.Get(2)
|
|
_ = foo.Get(3) // stack trace should mention this line
|
|
}
|