mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
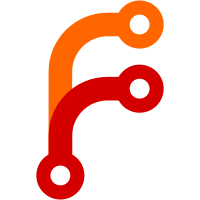
At VARKILLs, zero a variable if it is ambiguously live. After the VARKILL anything this variable references might be collected. If it were to become live again later, the GC will see references to already-collected objects. We don't know a variable is ambiguously live until very late in compilation (after lowering, register allocation, ...), so it is hard to generate the code in an arch-independent way. We also have to be careful not to clobber any registers. Fortunately, this almost never happens so performance is ~irrelevant. There are only 2 instances where this triggers in the stdlib. Fixes #20029 Change-Id: Ia9585a91d7b823fad4a9d141d954464cc7af31f4 Reviewed-on: https://go-review.googlesource.com/41076 Run-TryBot: Keith Randall <khr@golang.org> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: David Chase <drchase@google.com>
33 lines
583 B
Go
33 lines
583 B
Go
// run
|
|
|
|
// Copyright 2017 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Issue 20029: make sure we zero at VARKILLs of
|
|
// ambiguously live variables.
|
|
// The ambiguously live variable here is the hiter
|
|
// for the inner range loop.
|
|
|
|
package main
|
|
|
|
import "runtime"
|
|
|
|
func f(m map[int]int) {
|
|
outer:
|
|
for i := 0; i < 10; i++ {
|
|
for k := range m {
|
|
if k == 5 {
|
|
continue outer
|
|
}
|
|
}
|
|
runtime.GC()
|
|
break
|
|
}
|
|
runtime.GC()
|
|
}
|
|
func main() {
|
|
m := map[int]int{1: 2, 2: 3, 3: 4}
|
|
f(m)
|
|
}
|