mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
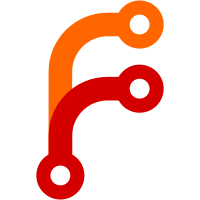
This CL fixes two issues: 1. Load ops were initially always lowered to unsigned loads, even for signed types. This was fine by itself however LoadReg ops (used to re-load spilled values) were lowered to signed loads for signed types. This meant that spills could invalidate optimizations that assumed the original unsigned load. 2. Types were not always being maintained correctly through rules designed to eliminate unnecessary zero and sign extensions. Fixes #18906. Change-Id: I95785dcadba03f7e3e94524677e7d8d3d3b9b737 Reviewed-on: https://go-review.googlesource.com/36256 Run-TryBot: Michael Munday <munday@ca.ibm.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Cherry Zhang <cherryyz@google.com>
37 lines
526 B
Go
37 lines
526 B
Go
// run
|
|
|
|
// Copyright 2017 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
//go:noinline
|
|
func f(x int) {
|
|
}
|
|
|
|
//go:noinline
|
|
func val() int8 {
|
|
return -1
|
|
}
|
|
|
|
var (
|
|
array = [257]int{}
|
|
slice = array[1:]
|
|
)
|
|
|
|
func init() {
|
|
for i := range array {
|
|
array[i] = i - 1
|
|
}
|
|
}
|
|
|
|
func main() {
|
|
x := val()
|
|
y := int(uint8(x))
|
|
f(y) // try and force y to be calculated and spilled
|
|
if slice[y] != 255 {
|
|
panic("incorrect value")
|
|
}
|
|
}
|