mirror of
https://github.com/golang/go
synced 2024-07-21 01:55:06 +00:00
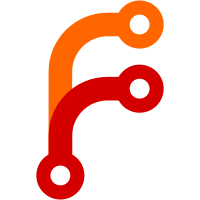
When nilcheck runs, the values in a block are not in any particular order. So any facts derived from examining the blocks shouldn't be used until we reach the next block. This is suboptimal as it won't eliminate nil checks within a block. But it's probably a better fix for now as it is a much smaller change than other strategies for fixing this bug. nilptr3.go changes are mostly because for this pattern: _ = *p _ = *p either nil check is fine to keep, and this CL changes which one the compiler tends to keep. There are a few regressions from code like this: _ = *p f() _ = *p For this pattern, after this CL we issue 2 nil checks instead of one. (For the curious, this happens because intra-block nil check elimination now falls to CSE, not nilcheck proper. The former pattern has two nil checks with the same store argument. The latter pattern has two nil checks with different store arguments.) Fixes #18725 Change-Id: I3721b494c8bc9ba1142dc5c4361ea55c66920ac8 Reviewed-on: https://go-review.googlesource.com/35485 Reviewed-by: Cherry Zhang <cherryyz@google.com>
25 lines
415 B
Go
25 lines
415 B
Go
// run
|
|
|
|
// Copyright 2017 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import "os"
|
|
|
|
func panicWhenNot(cond bool) {
|
|
if cond {
|
|
os.Exit(0)
|
|
} else {
|
|
panic("nilcheck elim failed")
|
|
}
|
|
}
|
|
|
|
func main() {
|
|
e := (*string)(nil)
|
|
panicWhenNot(e == e)
|
|
// Should never reach this line.
|
|
panicWhenNot(*e == *e)
|
|
}
|