mirror of
https://github.com/golang/go
synced 2024-07-19 19:44:12 +00:00
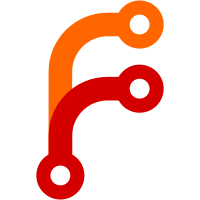
CL 4313064 fixed its test case but did not address a general enough problem: type T1 struct { F *T2 } type T2 T1 type T3 T2 could still end up copying the definition of T1 for T2 before T1 was done being evaluated, or T3 before T2 was done. In order to propagate the updates correctly, record a copy of an incomplete type for re-execution once the type is completed. Roll back CL 4313064. Fixes #3709. R=ken2 CC=golang-dev, lstoakes https://golang.org/cl/6301059
63 lines
1.2 KiB
Go
63 lines
1.2 KiB
Go
// errorcheck
|
|
|
|
// Copyright 2011 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test map declarations of many types, including erroneous ones.
|
|
// Does not compile.
|
|
|
|
package main
|
|
|
|
func main() {}
|
|
|
|
type v bool
|
|
|
|
var (
|
|
// valid
|
|
_ map[int8]v
|
|
_ map[uint8]v
|
|
_ map[int16]v
|
|
_ map[uint16]v
|
|
_ map[int32]v
|
|
_ map[uint32]v
|
|
_ map[int64]v
|
|
_ map[uint64]v
|
|
_ map[int]v
|
|
_ map[uint]v
|
|
_ map[uintptr]v
|
|
_ map[float32]v
|
|
_ map[float64]v
|
|
_ map[complex64]v
|
|
_ map[complex128]v
|
|
_ map[bool]v
|
|
_ map[string]v
|
|
_ map[chan int]v
|
|
_ map[*int]v
|
|
_ map[struct{}]v
|
|
_ map[[10]int]v
|
|
|
|
// invalid
|
|
_ map[[]int]v // ERROR "invalid map key"
|
|
_ map[func()]v // ERROR "invalid map key"
|
|
_ map[map[int]int]v // ERROR "invalid map key"
|
|
_ map[T1]v // ERROR "invalid map key"
|
|
_ map[T2]v // ERROR "invalid map key"
|
|
_ map[T3]v // ERROR "invalid map key"
|
|
_ map[T4]v // ERROR "invalid map key"
|
|
_ map[T5]v
|
|
_ map[T6]v
|
|
_ map[T7]v
|
|
_ map[T8]v
|
|
)
|
|
|
|
type T1 []int
|
|
type T2 struct { F T1 }
|
|
type T3 []T4
|
|
type T4 struct { F T3 }
|
|
|
|
type T5 *int
|
|
type T6 struct { F T5 }
|
|
type T7 *T4
|
|
type T8 struct { F *T7 }
|