mirror of
https://github.com/golang/go
synced 2024-07-19 19:44:12 +00:00
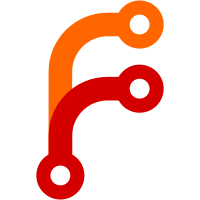
Previously merely printing an error would cause the golden file comparison (in 'bash run') to fail, but that is no longer the case with the new run.go driver. R=iant CC=golang-dev https://golang.org/cl/7310087
63 lines
1.2 KiB
Go
63 lines
1.2 KiB
Go
// run
|
|
|
|
// Copyright 2011 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test divide corner cases.
|
|
|
|
package main
|
|
|
|
import "fmt"
|
|
|
|
func f8(x, y, q, r int8) {
|
|
if t := x / y; t != q {
|
|
fmt.Printf("%d/%d = %d, want %d\n", x, y, t, q)
|
|
panic("divide")
|
|
}
|
|
if t := x % y; t != r {
|
|
fmt.Printf("%d%%%d = %d, want %d\n", x, y, t, r)
|
|
panic("divide")
|
|
}
|
|
}
|
|
|
|
func f16(x, y, q, r int16) {
|
|
if t := x / y; t != q {
|
|
fmt.Printf("%d/%d = %d, want %d\n", x, y, t, q)
|
|
panic("divide")
|
|
}
|
|
if t := x % y; t != r {
|
|
fmt.Printf("%d%%%d = %d, want %d\n", x, y, t, r)
|
|
panic("divide")
|
|
}
|
|
}
|
|
|
|
func f32(x, y, q, r int32) {
|
|
if t := x / y; t != q {
|
|
fmt.Printf("%d/%d = %d, want %d\n", x, y, t, q)
|
|
panic("divide")
|
|
}
|
|
if t := x % y; t != r {
|
|
fmt.Printf("%d%%%d = %d, want %d\n", x, y, t, r)
|
|
panic("divide")
|
|
}
|
|
}
|
|
|
|
func f64(x, y, q, r int64) {
|
|
if t := x / y; t != q {
|
|
fmt.Printf("%d/%d = %d, want %d\n", x, y, t, q)
|
|
panic("divide")
|
|
}
|
|
if t := x % y; t != r {
|
|
fmt.Printf("%d%%%d = %d, want %d\n", x, y, t, r)
|
|
panic("divide")
|
|
}
|
|
}
|
|
|
|
func main() {
|
|
f8(-1<<7, -1, -1<<7, 0)
|
|
f16(-1<<15, -1, -1<<15, 0)
|
|
f32(-1<<31, -1, -1<<31, 0)
|
|
f64(-1<<63, -1, -1<<63, 0)
|
|
}
|