mirror of
https://github.com/golang/go
synced 2024-11-02 11:50:30 +00:00
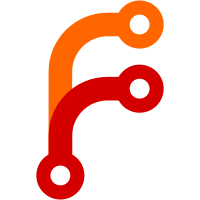
Follows suit with https://go-review.googlesource.com/#/c/20111. Generated by running $ grep -R 'Go Authors. All' * | cut -d":" -f1 | while read F;do perl -pi -e 's/Go Authors. All/Go Authors. All/g' $F;done The code in cmd/internal/unvendor wasn't changed. Fixes #15213 Change-Id: I4f235cee0a62ec435f9e8540a1ec08ae03b1a75f Reviewed-on: https://go-review.googlesource.com/21819 Reviewed-by: Ian Lance Taylor <iant@golang.org> Run-TryBot: Ian Lance Taylor <iant@golang.org> TryBot-Result: Gobot Gobot <gobot@golang.org>
85 lines
1.2 KiB
Go
85 lines
1.2 KiB
Go
// run
|
|
|
|
// Copyright 2010 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test of recover for run-time errors.
|
|
|
|
// TODO(rsc):
|
|
// null pointer accesses
|
|
|
|
package main
|
|
|
|
import "strings"
|
|
|
|
var x = make([]byte, 10)
|
|
|
|
func main() {
|
|
test1()
|
|
test2()
|
|
test3()
|
|
test4()
|
|
test5()
|
|
test6()
|
|
test7()
|
|
}
|
|
|
|
func mustRecover(s string) {
|
|
v := recover()
|
|
if v == nil {
|
|
panic("expected panic")
|
|
}
|
|
if e := v.(error).Error(); strings.Index(e, s) < 0 {
|
|
panic("want: " + s + "; have: " + e)
|
|
}
|
|
}
|
|
|
|
func test1() {
|
|
defer mustRecover("index")
|
|
println(x[123])
|
|
}
|
|
|
|
func test2() {
|
|
defer mustRecover("slice")
|
|
println(x[5:15])
|
|
}
|
|
|
|
func test3() {
|
|
defer mustRecover("slice")
|
|
var lo = 11
|
|
var hi = 9
|
|
println(x[lo:hi])
|
|
}
|
|
|
|
func test4() {
|
|
defer mustRecover("interface")
|
|
var x interface{} = 1
|
|
println(x.(float32))
|
|
}
|
|
|
|
type T struct {
|
|
a, b int
|
|
c []int
|
|
}
|
|
|
|
func test5() {
|
|
defer mustRecover("uncomparable")
|
|
var x T
|
|
var z interface{} = x
|
|
println(z != z)
|
|
}
|
|
|
|
func test6() {
|
|
defer mustRecover("unhashable")
|
|
var x T
|
|
var z interface{} = x
|
|
m := make(map[interface{}]int)
|
|
m[z] = 1
|
|
}
|
|
|
|
func test7() {
|
|
defer mustRecover("divide by zero")
|
|
var x, y int
|
|
println(x / y)
|
|
}
|