mirror of
https://github.com/golang/go
synced 2024-11-02 11:50:30 +00:00
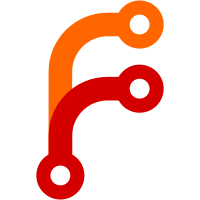
Support 'type C comparable' properly by using the same logic as for 'type T error', since ErrorType and ComparableType are entirely analogous. Added support for 'any' type as well, as requested by Robert. (For the future - we can't currently have 'any' anywhere other than in a constraint.) Fixes #47966 Change-Id: I68bd284ced9a8bfca7d2339cd576f3cb909b1b83 Reviewed-on: https://go-review.googlesource.com/c/go/+/345174 Trust: Dan Scales <danscales@google.com> Trust: Robert Griesemer <gri@golang.org> Run-TryBot: Dan Scales <danscales@google.com> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Robert Griesemer <gri@golang.org>
43 lines
856 B
Go
43 lines
856 B
Go
// run -gcflags=-G=3
|
|
|
|
// Copyright 2021 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test cases where a main dictionary is needed inside a generic function/method, because
|
|
// we are calling a method on a fully-instantiated type or a fully-instantiated function.
|
|
// (probably not common situations, of course)
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
)
|
|
|
|
type C comparable
|
|
|
|
type value[T C] struct {
|
|
val T
|
|
}
|
|
|
|
func (v *value[T]) test(def T) bool {
|
|
return (v.val == def)
|
|
}
|
|
|
|
func (v *value[T]) get(def T) T {
|
|
var c value[int]
|
|
if c.test(32) {
|
|
return def
|
|
} else if v.test(def) {
|
|
return def
|
|
} else {
|
|
return v.val
|
|
}
|
|
}
|
|
|
|
func main() {
|
|
var s value[string]
|
|
if got, want := s.get("ab"), ""; got != want {
|
|
panic(fmt.Sprintf("get() == %d, want %d", got, want))
|
|
}
|
|
}
|