mirror of
https://github.com/golang/go
synced 2024-09-04 23:44:16 +00:00
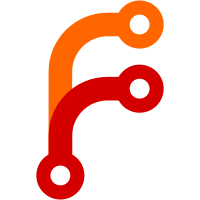
When collecting type parameters, wrap constraint literals of the form ~T or A|B into interfaces so the type checker doesn't have to deal with these type set expressions syntactically anywhere else but in interfaces (i.e., union types continue to appear only as embedded elements in interfaces). Since a type constraint doesn't need to be an interface anymore, we can remove the respective restriction. Instead, when accessing the constraint interface via TypeParam.iface, wrap non-interface constraints at that point and update the constraint so it happens only once. By computing the types sets of all type parameters at before the end of type-checking, we ensure that type constraints are in their final form when accessed through the API. For #48424. Change-Id: I3a47a644ad4ab20f91d93ee39fcf3214bb5a81f9 Reviewed-on: https://go-review.googlesource.com/c/go/+/353139 Trust: Robert Griesemer <gri@golang.org> Run-TryBot: Robert Griesemer <gri@golang.org> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Robert Findley <rfindley@google.com>
44 lines
1.1 KiB
Go
44 lines
1.1 KiB
Go
// errorcheck -G
|
|
|
|
// Copyright 2020 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Basic type parameter list type-checking (not syntax) errors.
|
|
|
|
package tparam1
|
|
|
|
// The predeclared identifier "any" may be used in place of interface{}.
|
|
var _ any
|
|
|
|
func _(_ any)
|
|
|
|
type _[_ any] struct{}
|
|
|
|
const N = 10
|
|
|
|
type (
|
|
_ []struct{} // slice
|
|
_ [N]struct{} // array
|
|
_[T any] struct{}
|
|
_[T, T any] struct{} // ERROR "T redeclared"
|
|
_[T1, T2 any, T3 any] struct{}
|
|
)
|
|
|
|
func _[T any]() {}
|
|
func _[T, T any]() {} // ERROR "T redeclared"
|
|
func _[T1, T2 any](x T1) T2 { panic(0) }
|
|
|
|
// Type parameters are visible from opening [ to end of function.
|
|
type C interface{}
|
|
|
|
func _[T interface{}]() {}
|
|
func _[T C]() {}
|
|
func _[T struct{}]() {} // ok if #48424 is accepted
|
|
func _[T interface{ m() T }]() {}
|
|
func _[T1 interface{ m() T2 }, T2 interface{ m() T1 }]() {
|
|
var _ T1
|
|
}
|
|
|
|
// TODO(gri) expand this
|