mirror of
https://github.com/golang/go
synced 2024-09-04 23:44:16 +00:00
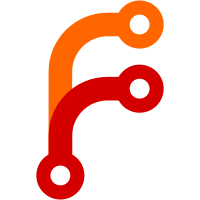
There were two main outer switch statements in node() that can just be combined. Also, for simplicity, changed an IsCmp() conditional into just another case in the switch statement. Also, the inner OCALL switch statement had a bunch of fairly duplicate cases. Combined the cases that all had no special semantics, into a single default case calling transformCall(). In the OCALL case in dictPass(), got rid of a check for OFUNCINST (which will always have been removed by this point). Also, eliminated an assert that could cause unneded failures. transformCall() should always be called if the node op is still OCALL, so no need to assert on the ops of call.X. Added an extra test in issue47078.go, to explicitly check for case where the X argument of a call is a DOTTYPE. Change-Id: Ifb3f812ce12820a4ce08afe2887f00f7fc00cd2f Reviewed-on: https://go-review.googlesource.com/c/go/+/358596 Trust: Dan Scales <danscales@google.com> Run-TryBot: Dan Scales <danscales@google.com> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Keith Randall <khr@golang.org>
57 lines
807 B
Go
57 lines
807 B
Go
// compile -G=3
|
|
|
|
// Copyright 2021 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
type Src1[T any] func() Src1[T]
|
|
|
|
func (s *Src1[T]) Next() {
|
|
*s = (*s)()
|
|
}
|
|
|
|
type Src2[T any] []func() Src2[T]
|
|
|
|
func (s Src2[T]) Next() {
|
|
_ = s[0]()
|
|
}
|
|
|
|
type Src3[T comparable] map[T]func() Src3[T]
|
|
|
|
func (s Src3[T]) Next() {
|
|
var a T
|
|
_ = s[a]()
|
|
}
|
|
|
|
type Src4[T any] chan func() T
|
|
|
|
func (s Src4[T]) Next() {
|
|
_ = (<-s)()
|
|
}
|
|
|
|
type Src5[T any] func() Src5[T]
|
|
|
|
func (s Src5[T]) Next() {
|
|
var x interface{} = s
|
|
_ = (x.(Src5[T]))()
|
|
}
|
|
|
|
func main() {
|
|
var src1 Src1[int]
|
|
src1.Next()
|
|
|
|
var src2 Src2[int]
|
|
src2.Next()
|
|
|
|
var src3 Src3[string]
|
|
src3.Next()
|
|
|
|
var src4 Src4[int]
|
|
src4.Next()
|
|
|
|
var src5 Src5[int]
|
|
src5.Next()
|
|
}
|