mirror of
https://github.com/golang/go
synced 2024-07-20 09:44:05 +00:00
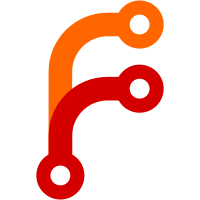
This commit adapts compile tool to create correct nilchecks for AIX. AIX allows to load a nil pointer. Therefore, the default nilcheck which issues a load must be replaced by a CMP instruction followed by a store at 0x0 if the value is nil. The store will trigger a SIGSEGV as on others OS. The nilcheck algorithm must be adapted to do not remove nilcheck if it's only a read. Stores are detected with v.Type.IsMemory(). Tests related to nilptr must be adapted to the previous changements. nilptr.go cannot be used as it's because the AIX address space starts at 1<<32. Change-Id: I9f5aaf0b7e185d736a9b119c0ed2fe4e5bd1e7af Reviewed-on: https://go-review.googlesource.com/c/144538 Run-TryBot: Tobias Klauser <tobias.klauser@gmail.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Keith Randall <khr@golang.org>
33 lines
812 B
Go
33 lines
812 B
Go
// errorcheck -0 -d=nil
|
|
|
|
// +build wasm
|
|
|
|
// Copyright 2018 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test that nil checks are removed.
|
|
// Optimization is enabled.
|
|
|
|
package p
|
|
|
|
func f5(p *float32, q *float64, r *float32, s *float64) float64 {
|
|
x := float64(*p) // ERROR "generated nil check"
|
|
y := *q // ERROR "generated nil check"
|
|
*r = 7 // ERROR "generated nil check"
|
|
*s = 9 // ERROR "generated nil check"
|
|
return x + y
|
|
}
|
|
|
|
type T [29]byte
|
|
|
|
func f6(p, q *T) {
|
|
x := *p // ERROR "generated nil check"
|
|
*q = x // ERROR "generated nil check"
|
|
}
|
|
|
|
// make sure to remove nil check for memory move (issue #18003)
|
|
func f8(t *[8]int) [8]int {
|
|
return *t // ERROR "generated nil check"
|
|
}
|