mirror of
https://github.com/golang/go
synced 2024-07-20 09:44:05 +00:00
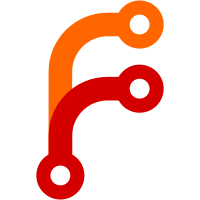
In general, a conversion to interface type may require values to be boxed, which in turn necessitates escape analysis to determine whether the boxed representation can be stack allocated. However, esc.go used to unconditionally print escape analysis decisions about OCONVIFACE, even for conversions that don't require boxing (e.g., pointers, channels, maps, functions). For test compatibility with esc.go, escape.go similarly printed these useless diagnostics. This CL removes the diagnostics, and updates test expectations accordingly. Change-Id: I97c57a4a08e44d265bba516c78426ff4f2bf1e12 Reviewed-on: https://go-review.googlesource.com/c/go/+/192697 Run-TryBot: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: Cherry Zhang <cherryyz@google.com>
70 lines
2.2 KiB
Go
70 lines
2.2 KiB
Go
// errorcheck -0 -m -l
|
|
|
|
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Test escape analysis for unsafe.Pointer rules.
|
|
|
|
package escape
|
|
|
|
import (
|
|
"reflect"
|
|
"unsafe"
|
|
)
|
|
|
|
// (1) Conversion of a *T1 to Pointer to *T2.
|
|
|
|
func convert(p *float64) *uint64 { // ERROR "leaking param: p to result ~r1 level=0$"
|
|
return (*uint64)(unsafe.Pointer(p))
|
|
}
|
|
|
|
// (3) Conversion of a Pointer to a uintptr and back, with arithmetic.
|
|
|
|
func arithAdd() unsafe.Pointer {
|
|
var x [2]byte // ERROR "moved to heap: x"
|
|
return unsafe.Pointer(uintptr(unsafe.Pointer(&x[0])) + 1)
|
|
}
|
|
|
|
func arithSub() unsafe.Pointer {
|
|
var x [2]byte // ERROR "moved to heap: x"
|
|
return unsafe.Pointer(uintptr(unsafe.Pointer(&x[1])) - 1)
|
|
}
|
|
|
|
func arithMask() unsafe.Pointer {
|
|
var x [2]byte // ERROR "moved to heap: x"
|
|
return unsafe.Pointer(uintptr(unsafe.Pointer(&x[1])) &^ 1)
|
|
}
|
|
|
|
// (5) Conversion of the result of reflect.Value.Pointer or
|
|
// reflect.Value.UnsafeAddr from uintptr to Pointer.
|
|
|
|
// BAD: should be "leaking param: p to result ~r1 level=0$"
|
|
func valuePointer(p *int) unsafe.Pointer { // ERROR "leaking param: p$"
|
|
return unsafe.Pointer(reflect.ValueOf(p).Pointer())
|
|
}
|
|
|
|
// BAD: should be "leaking param: p to result ~r1 level=0$"
|
|
func valueUnsafeAddr(p *int) unsafe.Pointer { // ERROR "leaking param: p$"
|
|
return unsafe.Pointer(reflect.ValueOf(p).Elem().UnsafeAddr())
|
|
}
|
|
|
|
// (6) Conversion of a reflect.SliceHeader or reflect.StringHeader
|
|
// Data field to or from Pointer.
|
|
|
|
func fromSliceData(s []int) unsafe.Pointer { // ERROR "leaking param: s to result ~r1 level=0$"
|
|
return unsafe.Pointer((*reflect.SliceHeader)(unsafe.Pointer(&s)).Data)
|
|
}
|
|
|
|
func fromStringData(s string) unsafe.Pointer { // ERROR "leaking param: s to result ~r1 level=0$"
|
|
return unsafe.Pointer((*reflect.StringHeader)(unsafe.Pointer(&s)).Data)
|
|
}
|
|
|
|
func toSliceData(s *[]int, p unsafe.Pointer) { // ERROR "s does not escape" "leaking param: p$"
|
|
(*reflect.SliceHeader)(unsafe.Pointer(s)).Data = uintptr(p)
|
|
}
|
|
|
|
func toStringData(s *string, p unsafe.Pointer) { // ERROR "s does not escape" "leaking param: p$"
|
|
(*reflect.SliceHeader)(unsafe.Pointer(s)).Data = uintptr(p)
|
|
}
|