mirror of
https://github.com/golang/go
synced 2024-11-05 18:36:08 +00:00
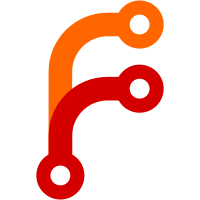
The next CL will remove the -G flag, effectively hard-coding it to its current default (-G=3). Change-Id: Ib4743b529206928f9f1cca9fdb19989728327831 Reviewed-on: https://go-review.googlesource.com/c/go/+/388534 Reviewed-by: Keith Randall <khr@golang.org> Trust: Matthew Dempsky <mdempsky@google.com> Run-TryBot: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Gopher Robot <gobot@golang.org>
65 lines
1.1 KiB
Go
65 lines
1.1 KiB
Go
// run
|
|
|
|
// Copyright 2022 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
)
|
|
|
|
func main() {
|
|
x := [][]int{{1}}
|
|
y := [][]int{{2, 3}}
|
|
IntersectSS(x, y)
|
|
}
|
|
|
|
type list[E any] interface {
|
|
~[]E
|
|
Equal(x, y E) bool
|
|
}
|
|
|
|
// ss is a set of sets
|
|
type ss[E comparable, T []E] []T
|
|
|
|
func (ss[E, T]) Equal(a, b T) bool {
|
|
return SetEq(a, b)
|
|
}
|
|
|
|
func IntersectSS[E comparable](x, y [][]E) [][]E {
|
|
return IntersectT[[]E, ss[E, []E]](ss[E, []E](x), ss[E, []E](y))
|
|
}
|
|
|
|
func IntersectT[E any, L list[E]](x, y L) L {
|
|
var z L
|
|
outer:
|
|
for _, xe := range x {
|
|
fmt.Println("xe", xe)
|
|
for _, ye := range y {
|
|
fmt.Println("ye", ye)
|
|
fmt.Println("x", x)
|
|
if x.Equal(xe, ye) {
|
|
fmt.Println("appending")
|
|
z = append(z, xe)
|
|
continue outer
|
|
}
|
|
}
|
|
}
|
|
return z
|
|
}
|
|
|
|
func SetEq[S []E, E comparable](x, y S) bool {
|
|
fmt.Println("SetEq", x, y)
|
|
outer:
|
|
for _, xe := range x {
|
|
for _, ye := range y {
|
|
if xe == ye {
|
|
continue outer
|
|
}
|
|
}
|
|
return false // xs wasn't found in y
|
|
}
|
|
return true
|
|
}
|