mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
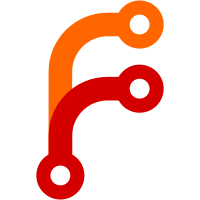
Change stencil.go:getDictionaryValue() and reflect.go:getDictionary() to reuse any existing name node that has been created for the needed global dictionary. Otherwise, these functions may set the Def on a specific dictionary sym to two different name nodes, which means the first node will not satisfy the invariant 'n.Sym().Def.(*ir.Name) == n' (which is the assertion in this issue). Fixes #47896 Change-Id: I1e7ae1efd077a83c7878b4342feb6d28d52476cc Reviewed-on: https://go-review.googlesource.com/c/go/+/344609 Run-TryBot: Dan Scales <danscales@google.com> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Keith Randall <khr@golang.org> Trust: Dan Scales <danscales@google.com>
74 lines
1.4 KiB
Go
74 lines
1.4 KiB
Go
// compile -G=3
|
|
|
|
// Copyright 2021 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import (
|
|
"database/sql"
|
|
)
|
|
|
|
// Collection generic interface which things can be added to.
|
|
type Collection[T any] interface {
|
|
Add(T)
|
|
}
|
|
|
|
// Slice generic slice implementation of a Collection
|
|
type Slice[T any] []*T
|
|
|
|
func (s *Slice[T]) Add(t *T) {
|
|
*s = append(*s, t)
|
|
}
|
|
|
|
type Scanner interface {
|
|
Scan(...interface{}) error
|
|
}
|
|
|
|
type Mapper[T any] func(s Scanner, t T) error
|
|
|
|
type Repository[T any] struct {
|
|
db *sql.DB
|
|
}
|
|
|
|
func (r *Repository[T]) scan(rows *sql.Rows, m Mapper[*T], c Collection[*T]) error {
|
|
for rows.Next() {
|
|
t := new(T)
|
|
if err := m(rows, t); err != nil {
|
|
return err
|
|
}
|
|
c.Add(t)
|
|
}
|
|
return rows.Err()
|
|
}
|
|
|
|
func (r *Repository[T]) query(query string, m Mapper[*T], c Collection[*T]) error {
|
|
rows, err := r.db.Query(query)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if err := r.scan(rows, m, c); err != nil {
|
|
rows.Close()
|
|
return err
|
|
}
|
|
return rows.Close()
|
|
}
|
|
|
|
type Actor struct {
|
|
ActorID uint16
|
|
FirstName string
|
|
LastName string
|
|
}
|
|
|
|
type ActorRepository struct {
|
|
r Repository[Actor]
|
|
}
|
|
|
|
func (ActorRepository) scan(s Scanner, a *Actor) error {
|
|
return s.Scan(&a.ActorID, &a.FirstName, &a.LastName)
|
|
}
|
|
|
|
func (r *ActorRepository) SelectAll(c Collection[*Actor]) error {
|
|
return r.r.query("SELECT `actor_id`, `first_name`, `last_name` FROM `actor` LIMIT 10", r.scan, c)
|
|
}
|