mirror of
https://github.com/golang/go
synced 2024-09-15 22:20:06 +00:00
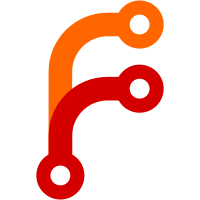
Currently we only include static entries in the hint for sizing the map when allocating a map for a map literal. Change that to include all entries. This will be an overallocation if the dynamic entries in the map have equal keys, but equal keys in map literals are rare, and at worst we waste a bit of space. Fixes #43020 Change-Id: I232f82f15316bdf4ea6d657d25a0b094b77884ce Reviewed-on: https://go-review.googlesource.com/c/go/+/383634 Run-TryBot: Keith Randall <khr@golang.org> Trust: Keith Randall <khr@golang.org> Trust: Josh Bleecher Snyder <josharian@gmail.com> Reviewed-by: Josh Bleecher Snyder <josharian@gmail.com> TryBot-Result: Gopher Robot <gobot@golang.org>
155 lines
3.1 KiB
Go
155 lines
3.1 KiB
Go
// asmcheck
|
|
|
|
// Copyright 2018 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package codegen
|
|
|
|
// This file contains code generation tests related to the handling of
|
|
// map types.
|
|
|
|
// ------------------- //
|
|
// Access Const //
|
|
// ------------------- //
|
|
|
|
// Direct use of constants in fast map access calls (Issue #19015).
|
|
|
|
func AccessInt1(m map[int]int) int {
|
|
// amd64:"MOV[LQ]\t[$]5"
|
|
return m[5]
|
|
}
|
|
|
|
func AccessInt2(m map[int]int) bool {
|
|
// amd64:"MOV[LQ]\t[$]5"
|
|
_, ok := m[5]
|
|
return ok
|
|
}
|
|
|
|
func AccessString1(m map[string]int) int {
|
|
// amd64:`.*"abc"`
|
|
return m["abc"]
|
|
}
|
|
|
|
func AccessString2(m map[string]int) bool {
|
|
// amd64:`.*"abc"`
|
|
_, ok := m["abc"]
|
|
return ok
|
|
}
|
|
|
|
// ------------------- //
|
|
// String Conversion //
|
|
// ------------------- //
|
|
|
|
func LookupStringConversionSimple(m map[string]int, bytes []byte) int {
|
|
// amd64:-`.*runtime\.slicebytetostring\(`
|
|
return m[string(bytes)]
|
|
}
|
|
|
|
func LookupStringConversionStructLit(m map[struct{ string }]int, bytes []byte) int {
|
|
// amd64:-`.*runtime\.slicebytetostring\(`
|
|
return m[struct{ string }{string(bytes)}]
|
|
}
|
|
|
|
func LookupStringConversionArrayLit(m map[[2]string]int, bytes []byte) int {
|
|
// amd64:-`.*runtime\.slicebytetostring\(`
|
|
return m[[2]string{string(bytes), string(bytes)}]
|
|
}
|
|
|
|
func LookupStringConversionNestedLit(m map[[1]struct{ s [1]string }]int, bytes []byte) int {
|
|
// amd64:-`.*runtime\.slicebytetostring\(`
|
|
return m[[1]struct{ s [1]string }{struct{ s [1]string }{s: [1]string{string(bytes)}}}]
|
|
}
|
|
|
|
func LookupStringConversionKeyedArrayLit(m map[[2]string]int, bytes []byte) int {
|
|
// amd64:-`.*runtime\.slicebytetostring\(`
|
|
return m[[2]string{0: string(bytes)}]
|
|
}
|
|
|
|
// ------------------- //
|
|
// Map Clear //
|
|
// ------------------- //
|
|
|
|
// Optimization of map clear idiom (Issue #20138).
|
|
|
|
func MapClearReflexive(m map[int]int) {
|
|
// amd64:`.*runtime\.mapclear`
|
|
// amd64:-`.*runtime\.mapiterinit`
|
|
for k := range m {
|
|
delete(m, k)
|
|
}
|
|
}
|
|
|
|
func MapClearIndirect(m map[int]int) {
|
|
s := struct{ m map[int]int }{m: m}
|
|
// amd64:`.*runtime\.mapclear`
|
|
// amd64:-`.*runtime\.mapiterinit`
|
|
for k := range s.m {
|
|
delete(s.m, k)
|
|
}
|
|
}
|
|
|
|
func MapClearPointer(m map[*byte]int) {
|
|
// amd64:`.*runtime\.mapclear`
|
|
// amd64:-`.*runtime\.mapiterinit`
|
|
for k := range m {
|
|
delete(m, k)
|
|
}
|
|
}
|
|
|
|
func MapClearNotReflexive(m map[float64]int) {
|
|
// amd64:`.*runtime\.mapiterinit`
|
|
// amd64:-`.*runtime\.mapclear`
|
|
for k := range m {
|
|
delete(m, k)
|
|
}
|
|
}
|
|
|
|
func MapClearInterface(m map[interface{}]int) {
|
|
// amd64:`.*runtime\.mapiterinit`
|
|
// amd64:-`.*runtime\.mapclear`
|
|
for k := range m {
|
|
delete(m, k)
|
|
}
|
|
}
|
|
|
|
func MapClearSideEffect(m map[int]int) int {
|
|
k := 0
|
|
// amd64:`.*runtime\.mapiterinit`
|
|
// amd64:-`.*runtime\.mapclear`
|
|
for k = range m {
|
|
delete(m, k)
|
|
}
|
|
return k
|
|
}
|
|
|
|
func MapLiteralSizing(x int) (map[int]int, map[int]int) {
|
|
// amd64:"MOVL\t[$]10,"
|
|
m := map[int]int{
|
|
0: 0,
|
|
1: 1,
|
|
2: 2,
|
|
3: 3,
|
|
4: 4,
|
|
5: 5,
|
|
6: 6,
|
|
7: 7,
|
|
8: 8,
|
|
9: 9,
|
|
}
|
|
// amd64:"MOVL\t[$]10,"
|
|
n := map[int]int{
|
|
0: x,
|
|
1: x,
|
|
2: x,
|
|
3: x,
|
|
4: x,
|
|
5: x,
|
|
6: x,
|
|
7: x,
|
|
8: x,
|
|
9: x,
|
|
}
|
|
return m, n
|
|
}
|