mirror of
https://github.com/golang/go
synced 2024-10-04 15:09:59 +00:00
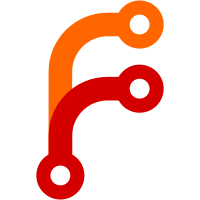
Since BCE happens over several passes (opt, loopbce, prove) it's easy to regress especially with rewriting. The pass is only activated with special debug flag. Change-Id: I46205982e7a2751156db8e875d69af6138068f59 Reviewed-on: https://go-review.googlesource.com/21510 Run-TryBot: Alexandru Moșoi <alexandru@mosoi.ro> TryBot-Result: Gobot Gobot <gobot@golang.org> Reviewed-by: David Chase <drchase@google.com>
90 lines
1.4 KiB
Go
90 lines
1.4 KiB
Go
// +build amd64
|
|
// errorcheck -0 -d=ssa/check_bce/debug=3
|
|
|
|
package main
|
|
|
|
func f0(a []int) {
|
|
a[0] = 1 // ERROR "Found IsInBounds$"
|
|
a[0] = 1
|
|
a[6] = 1 // ERROR "Found IsInBounds$"
|
|
a[6] = 1
|
|
a[5] = 1
|
|
a[5] = 1
|
|
}
|
|
|
|
func f1(a [256]int, i int) {
|
|
useInt(a[i]) // ERROR "Found IsInBounds$"
|
|
useInt(a[i%256]) // ERROR "Found IsInBounds$"
|
|
useInt(a[i&255])
|
|
useInt(a[i&17])
|
|
|
|
if 4 <= i && i < len(a) {
|
|
useInt(a[i])
|
|
useInt(a[i-1]) // ERROR "Found IsInBounds$"
|
|
useInt(a[i-4]) // ERROR "Found IsInBounds$"
|
|
}
|
|
}
|
|
|
|
func f2(a [256]int, i uint) {
|
|
useInt(a[i]) // ERROR "Found IsInBounds$"
|
|
useInt(a[i%256])
|
|
useInt(a[i&255])
|
|
useInt(a[i&17])
|
|
}
|
|
|
|
func f3(a [256]int, i uint8) {
|
|
useInt(a[i])
|
|
useInt(a[i+10])
|
|
useInt(a[i+14])
|
|
}
|
|
|
|
func f4(a [27]int, i uint8) {
|
|
useInt(a[i%15])
|
|
useInt(a[i%19])
|
|
useInt(a[i%27])
|
|
}
|
|
|
|
func f5(a []int) {
|
|
if len(a) > 5 {
|
|
useInt(a[5])
|
|
useSlice(a[6:])
|
|
useSlice(a[:6]) // ERROR "Found IsSliceInBounds$"
|
|
}
|
|
}
|
|
|
|
func g1(a []int) {
|
|
for i := range a {
|
|
a[i] = i
|
|
useSlice(a[:i+1])
|
|
useSlice(a[:i])
|
|
}
|
|
}
|
|
|
|
func g2(a []int) {
|
|
useInt(a[3]) // ERROR "Found IsInBounds$"
|
|
useInt(a[2])
|
|
useInt(a[1])
|
|
useInt(a[0])
|
|
}
|
|
|
|
func g3(a []int) {
|
|
for i := range a[:256] { // ERROR "Found IsSliceInBounds$"
|
|
useInt(a[i]) // ERROR "Found IsInBounds$"
|
|
}
|
|
b := a[:256]
|
|
for i := range b {
|
|
useInt(b[i])
|
|
}
|
|
}
|
|
|
|
//go:noinline
|
|
func useInt(a int) {
|
|
}
|
|
|
|
//go:noinline
|
|
func useSlice(a []int) {
|
|
}
|
|
|
|
func main() {
|
|
}
|