mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
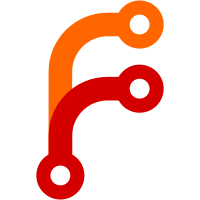
X ,s;^// \$G (\$D/)?\$F\.go *$;// compile;g X ,s;^// \$G (\$D/)?\$F\.go && \$L \$F\.\$A *$;// build;g X ,s;^// \$G (\$D/)?\$F\.go && \$L \$F\.\$A && \./\$A\.out *$;// run;g X ,s;^// errchk \$G( -e)? (\$D/)?\$F\.go *$;// errorcheck;g R=golang-dev CC=golang-dev https://golang.org/cl/5673078
54 lines
750 B
Go
54 lines
750 B
Go
// run
|
|
|
|
// Copyright 2009 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
type T struct {a, b int};
|
|
|
|
func println(x, y int) { }
|
|
|
|
func f(x interface{}) interface{} {
|
|
type T struct {a, b int};
|
|
|
|
if x == nil {
|
|
return T{2, 3};
|
|
}
|
|
|
|
t := x.(T);
|
|
println(t.a, t.b);
|
|
return x;
|
|
}
|
|
|
|
func main() {
|
|
inner_T := f(nil);
|
|
f(inner_T);
|
|
|
|
shouldPanic(p1)
|
|
}
|
|
|
|
func p1() {
|
|
outer_T := T{5, 7};
|
|
f(outer_T);
|
|
}
|
|
|
|
func shouldPanic(f func()) {
|
|
defer func() {
|
|
if recover() == nil {
|
|
panic("function should panic")
|
|
}
|
|
}()
|
|
f()
|
|
}
|
|
|
|
/*
|
|
This prints:
|
|
|
|
2 3
|
|
5 7
|
|
|
|
but it should crash: The type assertion on line 18 should fail
|
|
for the 2nd call to f with outer_T.
|
|
*/
|