mirror of
https://github.com/golang/go
synced 2024-11-02 13:42:29 +00:00
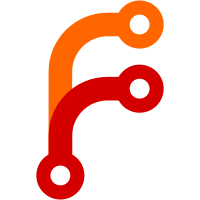
Some rules for PPC64 were checking for a case where a shift followed by an 'and' of a mask could be lowered, depending on the format of the mask. The function to verify if the mask was valid for this purpose was not checking if the mask was 0 which we don't want to allow. This case can happen if previous optimizations resulted in that mask value. This fixes isPPC64ValidShiftMask to check for a mask of 0 and return false. This also adds a codegen testcase to verify it doesn't try to match the rules in the future. Fixes #42610 Change-Id: I565d94e88495f51321ab365d6388c01e791b4dbb Reviewed-on: https://go-review.googlesource.com/c/go/+/270358 Run-TryBot: Lynn Boger <laboger@linux.vnet.ibm.com> TryBot-Result: Go Bot <gobot@golang.org> Reviewed-by: Paul Murphy <murp@ibm.com> Reviewed-by: Carlos Eduardo Seo <carlos.seo@linaro.org> Trust: Lynn Boger <laboger@linux.vnet.ibm.com>
30 lines
673 B
Go
30 lines
673 B
Go
// asmcheck
|
|
|
|
// Copyright 2020 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Don't allow 0 masks in shift lowering rules on ppc64x.
|
|
// See issue 42610.
|
|
|
|
package codegen
|
|
|
|
func f32(a []int32, i uint32) {
|
|
g := func(p int32) int32 {
|
|
i = uint32(p) * (uint32(p) & (i & 1))
|
|
return 1
|
|
}
|
|
// ppc64le: -"RLWNIM"
|
|
// ppc64: -"RLWNIM"
|
|
a[0] = g(8) >> 1
|
|
}
|
|
|
|
func f(a []int, i uint) {
|
|
g := func(p int) int {
|
|
i = uint(p) * (uint(p) & (i & 1))
|
|
return 1
|
|
}
|
|
// ppc64le: -"RLDIC"
|
|
// ppc64: -"RLDIC"
|
|
a[0] = g(8) >> 1
|
|
}
|