mirror of
https://github.com/golang/go
synced 2024-09-15 22:20:06 +00:00
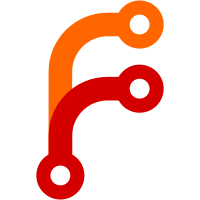
In CL 424734, I implemented pointer shaping for unified IR. Evidently though, we didn't have any test cases that check that uses of pointer-shaped expressions were handled correctly. In the reported test case, the struct field "children items[*node[T]]" gets shaped to "children items[go.shape.*uint8]" (underlying type "[]go.shape.*uint8"); and so the expression "n.children[i]" has type "go.shape.*uint8" and the ".items" field selection expression fails. The fix implemented in this CL is that any expression of derived type now gets an explicit "reshape" operation applied to it, to ensure it has the appropriate type for its context. E.g., the "n.children[i]" OINDEX expression above gets "reshaped" from "go.shape.*uint8" to "*node[go.shape.int]", allowing the field selection to succeed. This CL also adds a "-d=reshape" compiler debugging flag, because I anticipate debugging reshaping operations will be something to come up again in the future. Fixes #54535. Change-Id: Id847bd8f51300d2491d679505ee4d2e974ca972a Reviewed-on: https://go-review.googlesource.com/c/go/+/424936 Reviewed-by: David Chase <drchase@google.com> Reviewed-by: hopehook <hopehook@qq.com> Reviewed-by: Cuong Manh Le <cuong.manhle.vn@gmail.com> Run-TryBot: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Gopher Robot <gobot@golang.org>
38 lines
559 B
Go
38 lines
559 B
Go
// run
|
|
|
|
// Copyright 2022 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
type node[T any] struct {
|
|
items items[T]
|
|
children items[*node[T]]
|
|
}
|
|
|
|
func (n *node[T]) f(i int, j int) bool {
|
|
if len(n.children[i].items) < j {
|
|
return false
|
|
}
|
|
return true
|
|
}
|
|
|
|
type items[T any] []T
|
|
|
|
func main() {
|
|
_ = node[int]{}
|
|
_ = f[int]
|
|
}
|
|
|
|
type s[T, U any] struct {
|
|
a T
|
|
c U
|
|
}
|
|
|
|
func f[T any]() {
|
|
var x s[*struct{ b T }, *struct{ d int }]
|
|
_ = x.a.b
|
|
_ = x.c.d
|
|
}
|