mirror of
https://github.com/golang/go
synced 2024-07-20 17:48:31 +00:00
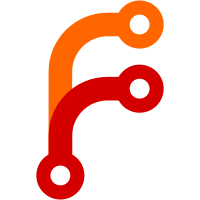
This allows more precision and matches types2's behavior. For backwards compatibility with gcimporter, for now we still need to write out declared constants as limited-precision floating-point values. To ensure consistent behavior of constant arithmetic whether it spans package boundaries or not, we include the full-precision rational representation in the compiler's extension section of the export data. Also, this CL simply uses the math/big.Rat.String text representation as the encoding. This is inefficient, but because it's only in the compiler's extension section, we can easily revisit this in the future. Declaring exported untyped float and complex constants isn't very common anyway. Within the standard library, only package math declares any at all, containing just 15. And those 15 are only imported a total of 12 times elsewhere in the standard library. Change-Id: I85ea23ab712e93fd3b68e52d60cbedce9be696a0 Reviewed-on: https://go-review.googlesource.com/c/go/+/286215 Run-TryBot: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Go Bot <gobot@golang.org> Trust: Matthew Dempsky <mdempsky@google.com> Trust: Robert Griesemer <gri@golang.org> Reviewed-by: Robert Griesemer <gri@golang.org>
48 lines
1.6 KiB
Go
48 lines
1.6 KiB
Go
// errorcheck
|
|
|
|
// Check flagging of invalid conversion of constant to float32/float64 near min/max boundaries.
|
|
|
|
// Copyright 2014 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
// See float_lit2.go for motivation for these values.
|
|
const (
|
|
two24 = 1.0 * (1 << 24)
|
|
two53 = 1.0 * (1 << 53)
|
|
two64 = 1.0 * (1 << 64)
|
|
two128 = two64 * two64
|
|
two256 = two128 * two128
|
|
two512 = two256 * two256
|
|
two768 = two512 * two256
|
|
two1024 = two512 * two512
|
|
|
|
ulp32 = two128 / two24
|
|
max32 = two128 - ulp32
|
|
|
|
ulp64 = two1024 / two53
|
|
max64 = two1024 - ulp64
|
|
)
|
|
|
|
var x = []interface{}{
|
|
float32(max32 + ulp32/2 - 1), // ok
|
|
float32(max32 + ulp32/2 - two128/two256), // ok
|
|
float32(max32 + ulp32/2), // ERROR "constant 3\.40282e\+38 overflows float32"
|
|
|
|
float32(-max32 - ulp32/2 + 1), // ok
|
|
float32(-max32 - ulp32/2 + two128/two256), // ok
|
|
float32(-max32 - ulp32/2), // ERROR "constant -3\.40282e\+38 overflows float32"
|
|
|
|
// If the compiler's internal floating point representation
|
|
// is shorter than 1024 bits, it cannot distinguish max64+ulp64/2-1 and max64+ulp64/2.
|
|
float64(max64 + ulp64/2 - two1024/two256), // ok
|
|
float64(max64 + ulp64/2 - 1), // ok
|
|
float64(max64 + ulp64/2), // ERROR "constant 1\.79769e\+308 overflows float64"
|
|
|
|
float64(-max64 - ulp64/2 + two1024/two256), // ok
|
|
float64(-max64 - ulp64/2 + 1), // ok
|
|
float64(-max64 - ulp64/2), // ERROR "constant -1\.79769e\+308 overflows float64"
|
|
}
|