mirror of
https://github.com/golang/go
synced 2024-09-04 23:44:16 +00:00
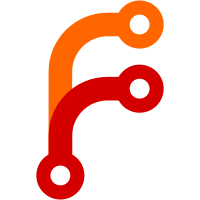
This CL moves two bits of related code from order.go to escape analysis: 1. The recognition of "unsafe uintptr" arguments passed to syscall-like functions. 2. The wrapping of go/defer function calls in parameter-free function literals. As with previous CLs, it would be nice to push this logic even further forward, but for now escape analysis seems most pragmatic. A couple side benefits: 1. It allows getting rid of the uintptrEscapesHack kludge. 2. When inserting wrappers, we can move some expressions into the wrapper and escape analyze them better. For example, the test expectation changes are all due to slice literals in go/defer calls where the slice is now constructed at the call site, and can now be stack allocated. Change-Id: I73679bcad7fa8d61d2fc52d4cea0dc5ff0de8c0c Reviewed-on: https://go-review.googlesource.com/c/go/+/330330 Run-TryBot: Matthew Dempsky <mdempsky@google.com> TryBot-Result: Go Bot <gobot@golang.org> Trust: Matthew Dempsky <mdempsky@google.com> Reviewed-by: Cuong Manh Le <cuong.manhle.vn@gmail.com>
50 lines
2.3 KiB
Go
50 lines
2.3 KiB
Go
// errorcheck -0 -m
|
|
|
|
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package p
|
|
|
|
func f(...*int) {} // ERROR "can inline f$"
|
|
|
|
func g() {
|
|
defer f()
|
|
defer f(new(int)) // ERROR "... argument does not escape$" "new\(int\) does not escape$"
|
|
defer f(new(int), new(int)) // ERROR "... argument does not escape$" "new\(int\) does not escape$"
|
|
|
|
defer f(nil...)
|
|
defer f([]*int{}...) // ERROR "\[\]\*int{} does not escape$"
|
|
defer f([]*int{new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) does not escape$"
|
|
defer f([]*int{new(int), new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) does not escape$"
|
|
|
|
go f()
|
|
go f(new(int)) // ERROR "... argument does not escape$" "new\(int\) escapes to heap$"
|
|
go f(new(int), new(int)) // ERROR "... argument does not escape$" "new\(int\) escapes to heap$"
|
|
|
|
go f(nil...)
|
|
go f([]*int{}...) // ERROR "\[\]\*int{} does not escape$"
|
|
go f([]*int{new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) escapes to heap$"
|
|
go f([]*int{new(int), new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) escapes to heap$"
|
|
|
|
for {
|
|
defer f()
|
|
defer f(new(int)) // ERROR "... argument does not escape$" "new\(int\) escapes to heap$"
|
|
defer f(new(int), new(int)) // ERROR "... argument does not escape$" "new\(int\) escapes to heap$"
|
|
|
|
defer f(nil...)
|
|
defer f([]*int{}...) // ERROR "\[\]\*int{} does not escape$"
|
|
defer f([]*int{new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) escapes to heap$"
|
|
defer f([]*int{new(int), new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) escapes to heap$"
|
|
|
|
go f()
|
|
go f(new(int)) // ERROR "... argument does not escape$" "new\(int\) escapes to heap$"
|
|
go f(new(int), new(int)) // ERROR "... argument does not escape$" "new\(int\) escapes to heap$"
|
|
|
|
go f(nil...)
|
|
go f([]*int{}...) // ERROR "\[\]\*int{} does not escape$"
|
|
go f([]*int{new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) escapes to heap$"
|
|
go f([]*int{new(int), new(int)}...) // ERROR "\[\]\*int{...} does not escape$" "new\(int\) escapes to heap$"
|
|
}
|
|
}
|