mirror of
https://github.com/dart-lang/sdk
synced 2024-09-16 00:29:48 +00:00
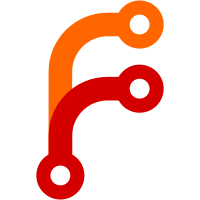
Right now most of the dart SDK's python is compatible with python2 or python3. This change fixes a few of the build scripts to make that completely true (at least when building the standard build on Linux). There are only four types of changes: - Bare `print` statements now use the `print ()` function - `commands.getoutput` becomes `subprocess.check_output` with `shell=True` - `xrange` becomes `range` - `print >> sys.stderr` becomes `sys.stderr.write` Starts work on addressing (but does not completely fix): https://github.com/dart-lang/sdk/issues/28793 See related issue: https://fuchsia-review.googlesource.com/c/fuchsia/+/272925 This change applys to both the `dev` and `master` branches. Change-Id: Ibd3eb9b1f57520d2d745f05c2ac430b1d20943da Closes #36662 https://github.com/dart-lang/sdk/pull/36662 GitOrigin-RevId: beab165294982a7e369daf6d61aea63efcab1b9b Change-Id: I6d240749a9ba0889b5a45a08f3c4c2c20291f484 Reviewed-on: https://dart-review.googlesource.com/c/sdk/+/99707 Reviewed-by: Alexander Thomas <athom@google.com> Commit-Queue: Alexander Thomas <athom@google.com>
49 lines
1.3 KiB
Python
49 lines
1.3 KiB
Python
# Downloads dill files from CIPD for each supported ABI version.
|
|
|
|
import os
|
|
import subprocess
|
|
import sys
|
|
import utils
|
|
|
|
|
|
def procWait(p):
|
|
while p.returncode is None:
|
|
p.communicate()
|
|
p.poll()
|
|
return p.returncode
|
|
|
|
|
|
def findAbiVersion(version):
|
|
cmd = ['cipd', 'instances', 'dart/abiversions/%d' % version]
|
|
p = subprocess.Popen(cmd,
|
|
stdout = subprocess.PIPE,
|
|
stderr = subprocess.PIPE,
|
|
shell = utils.IsWindows(),
|
|
cwd = utils.DART_DIR)
|
|
return procWait(p) == 0
|
|
|
|
|
|
def main():
|
|
abi_version = int(utils.GetAbiVersion())
|
|
oldest_abi_version = int(utils.GetOldestSupportedAbiVersion())
|
|
cmd = ['cipd', 'ensure', '-root', 'tools/abiversions', '-ensure-file', '-']
|
|
ensure_file = ''
|
|
for i in range(oldest_abi_version, abi_version + 1):
|
|
if findAbiVersion(i):
|
|
ensure_file += '@Subdir %d\ndart/abiversions/%d latest\n\n' % (i, i)
|
|
if not ensure_file:
|
|
return 0
|
|
p = subprocess.Popen(cmd,
|
|
stdin = subprocess.PIPE,
|
|
stdout = subprocess.PIPE,
|
|
stderr = subprocess.PIPE,
|
|
shell = utils.IsWindows(),
|
|
cwd = utils.DART_DIR)
|
|
p.communicate(ensure_file)
|
|
p.stdin.close()
|
|
return procWait(p)
|
|
|
|
|
|
if __name__ == '__main__':
|
|
sys.exit(main())
|